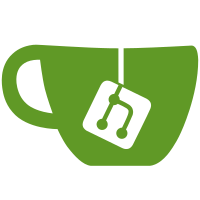
6 changed files with 135 additions and 19 deletions
@ -0,0 +1,85 @@ |
|||
import request from '@/utils/request'; |
|||
import type { ApiResult, PageResult } from '@/api'; |
|||
import type { Tenant, TenantParam } from './model/index'; |
|||
|
|||
/** |
|||
* 分页查询租户 |
|||
*/ |
|||
export async function pageTenant(params: TenantParam) { |
|||
const res = await request.get<ApiResult<PageResult<Tenant>>>('/tenant/page', { |
|||
params |
|||
}); |
|||
if (res.data.code === 0) { |
|||
return res.data.data; |
|||
} |
|||
return Promise.reject(new Error(res.data.message)); |
|||
} |
|||
|
|||
/** |
|||
* 查询租户列表 |
|||
*/ |
|||
export async function listTenant(params?: TenantParam) { |
|||
const res = await request.get<ApiResult<Tenant[]>>('/tenant', { |
|||
params |
|||
}); |
|||
if (res.data.code === 0 && res.data.data) { |
|||
return res.data.data; |
|||
} |
|||
return Promise.reject(new Error(res.data.message)); |
|||
} |
|||
|
|||
/** |
|||
* 添加租户 |
|||
*/ |
|||
export async function addTenant(data: Tenant) { |
|||
const res = await request.post<ApiResult<unknown>>('/tenant', data); |
|||
if (res.data.code === 0) { |
|||
return res.data.message; |
|||
} |
|||
return Promise.reject(new Error(res.data.message)); |
|||
} |
|||
|
|||
/** |
|||
* 修改租户 |
|||
*/ |
|||
export async function updateTenant(data: Tenant) { |
|||
const res = await request.put<ApiResult<unknown>>('/tenant', data); |
|||
if (res.data.code === 0) { |
|||
return res.data.message; |
|||
} |
|||
return Promise.reject(new Error(res.data.message)); |
|||
} |
|||
|
|||
/** |
|||
* 重置用户密码 |
|||
*/ |
|||
export async function updateTenantPassword( |
|||
tenantId?: number, |
|||
password = 'gxwebsoft.com' |
|||
) { |
|||
const res = await request.put<ApiResult<unknown>>('/tenant/password', { |
|||
tenantId, |
|||
password |
|||
}); |
|||
if (res.data.code === 0) { |
|||
return res.data.message; |
|||
} |
|||
return Promise.reject(new Error(res.data.message)); |
|||
} |
|||
|
|||
/** |
|||
* 检查IP是否存在 |
|||
*/ |
|||
export async function checkExistence( |
|||
field: string, |
|||
value: string, |
|||
id?: number |
|||
) { |
|||
const res = await request.get<ApiResult<unknown>>('/tenant/existence', { |
|||
params: { field, value, id } |
|||
}); |
|||
if (res.data.code === 0) { |
|||
return res.data.message; |
|||
} |
|||
return Promise.reject(new Error(res.data.message)); |
|||
} |
@ -0,0 +1,32 @@ |
|||
import type { PageParam } from '@/api'; |
|||
import { Company } from "@/api/system/company/model"; |
|||
|
|||
/** |
|||
* 租户 |
|||
*/ |
|||
export interface Tenant { |
|||
// 租户id
|
|||
tenantId?: number; |
|||
// 租户名称
|
|||
tenantName?: string; |
|||
// 客户ID
|
|||
customerId?: string; |
|||
// 备注
|
|||
comments?: string; |
|||
// 创建时间
|
|||
createTime?: string; |
|||
// 状态
|
|||
status?: string; |
|||
// 企业信息
|
|||
company?: Company; |
|||
// 配置信息
|
|||
config?: any; |
|||
} |
|||
|
|||
/** |
|||
* 租户搜索条件 |
|||
*/ |
|||
export interface TenantParam extends PageParam { |
|||
tenantName?: string; |
|||
customerId?: number; |
|||
} |
Loading…
Reference in new issue