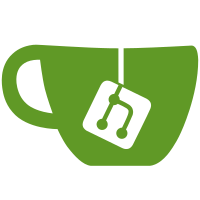
17 changed files with 1108 additions and 2 deletions
@ -0,0 +1,182 @@ |
|||
package com.gxwebsoft.tower.controller; |
|||
|
|||
import cn.hutool.core.date.DateTime; |
|||
import cn.hutool.core.date.DateUtil; |
|||
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
|||
import com.gxwebsoft.common.core.web.BaseController; |
|||
import com.gxwebsoft.common.system.entity.User; |
|||
import com.gxwebsoft.tower.entity.TowerProject; |
|||
import com.gxwebsoft.tower.service.TowerProjectService; |
|||
import com.gxwebsoft.tower.service.TowerSecurityService; |
|||
import com.gxwebsoft.tower.entity.TowerSecurity; |
|||
import com.gxwebsoft.tower.param.TowerSecurityParam; |
|||
import com.gxwebsoft.common.core.web.ApiResult; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import com.gxwebsoft.common.core.web.PageParam; |
|||
import com.gxwebsoft.common.core.web.BatchParam; |
|||
import com.gxwebsoft.common.core.annotation.OperationLog; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.apache.poi.ss.formula.functions.T; |
|||
import org.springframework.scheduling.annotation.Scheduled; |
|||
import org.springframework.security.access.prepost.PreAuthorize; |
|||
import org.springframework.transaction.annotation.Transactional; |
|||
import org.springframework.util.CollectionUtils; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import javax.annotation.Resource; |
|||
import java.util.Date; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 安全检查控制器 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-22 13:44:23 |
|||
*/ |
|||
@Api(tags = "安全检查管理") |
|||
@RestController |
|||
@RequestMapping("/api/tower/tower-security") |
|||
public class TowerSecurityController extends BaseController { |
|||
@Resource |
|||
private TowerSecurityService towerSecurityService; |
|||
@Resource |
|||
private TowerProjectService towerProjectService; |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:list')") |
|||
@OperationLog |
|||
@ApiOperation("分页查询安全检查") |
|||
@GetMapping("/page") |
|||
public ApiResult<PageResult<TowerSecurity>> page(TowerSecurityParam param) { |
|||
// PageParam<TowerSecurity, TowerSecurityParam> page = new PageParam<>(param);
|
|||
// page.setDefaultOrder("create_time desc");
|
|||
// return success(towerSecurityService.page(page, page.getWrapper()));
|
|||
// 使用关联查询
|
|||
return success(towerSecurityService.pageRel(param)); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:list')") |
|||
@OperationLog |
|||
@ApiOperation("查询全部安全检查") |
|||
@GetMapping() |
|||
public ApiResult<List<TowerSecurity>> list(TowerSecurityParam param) { |
|||
PageParam<TowerSecurity, TowerSecurityParam> page = new PageParam<>(param); |
|||
page.setDefaultOrder("create_time desc"); |
|||
return success(towerSecurityService.list(page.getOrderWrapper())); |
|||
// 使用关联查询
|
|||
//return success(towerSecurityService.listRel(param));
|
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:list')") |
|||
@OperationLog |
|||
@ApiOperation("根据id查询安全检查") |
|||
@GetMapping("/{id}") |
|||
public ApiResult<TowerSecurity> get(@PathVariable("id") Integer id) { |
|||
return success(towerSecurityService.getById(id)); |
|||
// 使用关联查询
|
|||
//return success(towerSecurityService.getByIdRel(id));
|
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:save')") |
|||
@OperationLog |
|||
@ApiOperation("添加安全检查") |
|||
@PostMapping() |
|||
public ApiResult<?> save(@RequestBody TowerSecurity towerSecurity) { |
|||
// 记录当前登录用户id
|
|||
User loginUser = getLoginUser(); |
|||
if (loginUser != null) { |
|||
towerSecurity.setUserId(loginUser.getUserId()); |
|||
} |
|||
// 生成编号
|
|||
DateTime date = DateUtil.date(); |
|||
final String code = DateUtil.format(date, "yyyyMMddHH"); |
|||
towerSecurity.setSecurityCode(code.concat(towerSecurity.getProjectId().toString())); |
|||
if (towerSecurityService.save(towerSecurity)) { |
|||
return success("添加成功"); |
|||
} |
|||
return fail("添加失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:update')") |
|||
@OperationLog |
|||
@ApiOperation("修改安全检查") |
|||
@PutMapping() |
|||
public ApiResult<?> update(@RequestBody TowerSecurity towerSecurity) { |
|||
if (towerSecurityService.updateById(towerSecurity)) { |
|||
return success("修改成功"); |
|||
} |
|||
return fail("修改失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:remove')") |
|||
@OperationLog |
|||
@ApiOperation("删除安全检查") |
|||
@DeleteMapping("/{id}") |
|||
public ApiResult<?> remove(@PathVariable("id") Integer id) { |
|||
if (towerSecurityService.removeById(id)) { |
|||
return success("删除成功"); |
|||
} |
|||
return fail("删除失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:save')") |
|||
@OperationLog |
|||
@ApiOperation("批量添加安全检查") |
|||
@PostMapping("/batch") |
|||
public ApiResult<?> saveBatch(@RequestBody List<TowerSecurity> list) { |
|||
if (towerSecurityService.saveBatch(list)) { |
|||
return success("添加成功"); |
|||
} |
|||
return fail("添加失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:update')") |
|||
@OperationLog |
|||
@ApiOperation("批量修改安全检查") |
|||
@PutMapping("/batch") |
|||
public ApiResult<?> removeBatch(@RequestBody BatchParam<TowerSecurity> batchParam) { |
|||
if (batchParam.update(towerSecurityService, "security_id")) { |
|||
return success("修改成功"); |
|||
} |
|||
return fail("修改失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:remove')") |
|||
@OperationLog |
|||
@ApiOperation("批量删除安全检查") |
|||
@DeleteMapping("/batch") |
|||
public ApiResult<?> removeBatch(@RequestBody List<Integer> ids) { |
|||
if (towerSecurityService.removeByIds(ids)) { |
|||
return success("删除成功"); |
|||
} |
|||
return fail("删除失败"); |
|||
} |
|||
|
|||
// @Scheduled(cron = "0 0 0 1 * ?")
|
|||
@Scheduled(fixedDelay = 5000, initialDelay = 5000) |
|||
@Transactional(rollbackFor = {Exception.class}) |
|||
public void wxAccountTask() { |
|||
System.out.println("任务:在建项目每月1号生产保养计划 = "); |
|||
final DateTime currentDate = DateUtil.date(); |
|||
int currentMonth = DateUtil.month(currentDate) + 1; |
|||
final List<TowerProject> list = towerProjectService.list(new LambdaQueryWrapper<TowerProject>().eq(TowerProject::getProjectStatus, "建设中").ne(TowerProject::getPlanMonth,currentMonth)); |
|||
System.out.println("list = " + list.size()); |
|||
if (!CollectionUtils.isEmpty(list)) { |
|||
list.forEach(d -> { |
|||
// 生成保养计划
|
|||
TowerSecurity towerSecurity = new TowerSecurity(); |
|||
towerSecurity.setProjectId(d.getProjectId()); |
|||
towerSecurity.setProjectName(d.getProjectName()); |
|||
towerSecurity.setAddress(d.getProjectAddress()); |
|||
towerSecurity.setTenantId(10049); |
|||
DateTime date = DateUtil.date(); |
|||
final String code = DateUtil.format(date, "yyyyMMdd"); |
|||
towerSecurity.setSecurityCode(code.concat(d.getProjectId().toString())); |
|||
d.setPlanMonth(currentMonth); |
|||
towerProjectService.updateById(d); |
|||
towerSecurityService.save(towerSecurity); |
|||
}); |
|||
} |
|||
} |
|||
|
|||
} |
@ -0,0 +1,139 @@ |
|||
package com.gxwebsoft.tower.controller; |
|||
|
|||
import com.gxwebsoft.common.core.web.BaseController; |
|||
import com.gxwebsoft.common.system.entity.User; |
|||
import com.gxwebsoft.tower.service.TowerSecurityRecordService; |
|||
import com.gxwebsoft.tower.entity.TowerSecurityRecord; |
|||
import com.gxwebsoft.tower.param.TowerSecurityRecordParam; |
|||
import com.gxwebsoft.common.core.web.ApiResult; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import com.gxwebsoft.common.core.web.PageParam; |
|||
import com.gxwebsoft.common.core.web.BatchParam; |
|||
import com.gxwebsoft.common.core.annotation.OperationLog; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.security.access.prepost.PreAuthorize; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import javax.annotation.Resource; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 保养记录控制器 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-22 13:44:23 |
|||
*/ |
|||
@Api(tags = "保养记录管理") |
|||
@RestController |
|||
@RequestMapping("/api/tower/tower-security-record") |
|||
public class TowerSecurityRecordController extends BaseController { |
|||
@Resource |
|||
private TowerSecurityRecordService towerSecurityRecordService; |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:list')") |
|||
@OperationLog |
|||
@ApiOperation("分页查询保养记录") |
|||
@GetMapping("/page") |
|||
public ApiResult<PageResult<TowerSecurityRecord>> page(TowerSecurityRecordParam param) { |
|||
// PageParam<TowerSecurityRecord, TowerSecurityRecordParam> page = new PageParam<>(param);
|
|||
// page.setDefaultOrder("create_time desc");
|
|||
// return success(towerSecurityRecordService.page(page, page.getWrapper()));
|
|||
// 使用关联查询
|
|||
return success(towerSecurityRecordService.pageRel(param)); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:list')") |
|||
@OperationLog |
|||
@ApiOperation("查询全部保养记录") |
|||
@GetMapping() |
|||
public ApiResult<List<TowerSecurityRecord>> list(TowerSecurityRecordParam param) { |
|||
PageParam<TowerSecurityRecord, TowerSecurityRecordParam> page = new PageParam<>(param); |
|||
page.setDefaultOrder("create_time desc"); |
|||
return success(towerSecurityRecordService.list(page.getOrderWrapper())); |
|||
// 使用关联查询
|
|||
//return success(towerSecurityRecordService.listRel(param));
|
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:list')") |
|||
@OperationLog |
|||
@ApiOperation("根据id查询保养记录") |
|||
@GetMapping("/{id}") |
|||
public ApiResult<TowerSecurityRecord> get(@PathVariable("id") Integer id) { |
|||
return success(towerSecurityRecordService.getById(id)); |
|||
// 使用关联查询
|
|||
//return success(towerSecurityRecordService.getByIdRel(id));
|
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:save')") |
|||
@OperationLog |
|||
@ApiOperation("添加保养记录") |
|||
@PostMapping() |
|||
public ApiResult<?> save(@RequestBody TowerSecurityRecord towerSecurityRecord) { |
|||
// 记录当前登录用户id
|
|||
User loginUser = getLoginUser(); |
|||
if (loginUser != null) { |
|||
towerSecurityRecord.setUserId(loginUser.getUserId()); |
|||
} |
|||
if (towerSecurityRecordService.save(towerSecurityRecord)) { |
|||
return success("添加成功"); |
|||
} |
|||
return fail("添加失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:update')") |
|||
@OperationLog |
|||
@ApiOperation("修改保养记录") |
|||
@PutMapping() |
|||
public ApiResult<?> update(@RequestBody TowerSecurityRecord towerSecurityRecord) { |
|||
if (towerSecurityRecordService.updateById(towerSecurityRecord)) { |
|||
return success("修改成功"); |
|||
} |
|||
return fail("修改失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:remove')") |
|||
@OperationLog |
|||
@ApiOperation("删除保养记录") |
|||
@DeleteMapping("/{id}") |
|||
public ApiResult<?> remove(@PathVariable("id") Integer id) { |
|||
if (towerSecurityRecordService.removeById(id)) { |
|||
return success("删除成功"); |
|||
} |
|||
return fail("删除失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:save')") |
|||
@OperationLog |
|||
@ApiOperation("批量添加保养记录") |
|||
@PostMapping("/batch") |
|||
public ApiResult<?> saveBatch(@RequestBody List<TowerSecurityRecord> list) { |
|||
if (towerSecurityRecordService.saveBatch(list)) { |
|||
return success("添加成功"); |
|||
} |
|||
return fail("添加失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:update')") |
|||
@OperationLog |
|||
@ApiOperation("批量修改保养记录") |
|||
@PutMapping("/batch") |
|||
public ApiResult<?> removeBatch(@RequestBody BatchParam<TowerSecurityRecord> batchParam) { |
|||
if (batchParam.update(towerSecurityRecordService, "security_id")) { |
|||
return success("修改成功"); |
|||
} |
|||
return fail("修改失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerSecurity:remove')") |
|||
@OperationLog |
|||
@ApiOperation("批量删除保养记录") |
|||
@DeleteMapping("/batch") |
|||
public ApiResult<?> removeBatch(@RequestBody List<Integer> ids) { |
|||
if (towerSecurityRecordService.removeByIds(ids)) { |
|||
return success("删除成功"); |
|||
} |
|||
return fail("删除失败"); |
|||
} |
|||
|
|||
} |
@ -0,0 +1,104 @@ |
|||
package com.gxwebsoft.tower.entity; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.IdType; |
|||
import com.baomidou.mybatisplus.annotation.TableId; |
|||
import java.time.LocalDateTime; |
|||
import com.baomidou.mybatisplus.annotation.TableLogic; |
|||
import java.io.Serializable; |
|||
import java.util.Date; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
import lombok.EqualsAndHashCode; |
|||
|
|||
/** |
|||
* 安全检查 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-22 13:44:23 |
|||
*/ |
|||
@Data |
|||
@EqualsAndHashCode(callSuper = false) |
|||
@ApiModel(value = "TowerSecurity对象", description = "安全检查") |
|||
public class TowerSecurity implements Serializable { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty(value = "自增ID") |
|||
@TableId(value = "security_id", type = IdType.AUTO) |
|||
private Integer securityId; |
|||
|
|||
@ApiModelProperty(value = "类型") |
|||
private Integer type; |
|||
|
|||
@ApiModelProperty(value = "项目名称") |
|||
private String projectName; |
|||
|
|||
@ApiModelProperty(value = "项目ID") |
|||
private Integer projectId; |
|||
|
|||
@ApiModelProperty(value = "保养编号") |
|||
private String securityCode; |
|||
|
|||
@ApiModelProperty(value = "所在地") |
|||
private String address; |
|||
|
|||
@ApiModelProperty(value = "经度") |
|||
private String longitude; |
|||
|
|||
@ApiModelProperty(value = "纬度") |
|||
private String latitude; |
|||
|
|||
@ApiModelProperty(value = "所在国家") |
|||
private String country; |
|||
|
|||
@ApiModelProperty(value = "所在省份") |
|||
private String province; |
|||
|
|||
@ApiModelProperty(value = "所在城市") |
|||
private String city; |
|||
|
|||
@ApiModelProperty(value = "所在辖区") |
|||
private String region; |
|||
|
|||
@ApiModelProperty(value = "区域名称") |
|||
private String regionName; |
|||
|
|||
@ApiModelProperty(value = "状态, 0正常, 1待修,2异常已修,3异常未修") |
|||
private Integer status; |
|||
|
|||
@ApiModelProperty(value = "备注") |
|||
private String comments; |
|||
|
|||
@ApiModelProperty(value = "问题描述") |
|||
private String problem; |
|||
|
|||
@ApiModelProperty(value = "处理方法") |
|||
private String processingMethod; |
|||
|
|||
@ApiModelProperty(value = "图片附件") |
|||
private String files; |
|||
|
|||
@ApiModelProperty(value = "是否更换配件") |
|||
private Boolean replaceAccessories; |
|||
|
|||
@ApiModelProperty(value = "排序号") |
|||
private Integer sortNumber; |
|||
|
|||
@ApiModelProperty(value = "所有人") |
|||
private Integer userId; |
|||
|
|||
@ApiModelProperty(value = "是否删除, 0否, 1是") |
|||
@TableLogic |
|||
private Integer deleted; |
|||
|
|||
@ApiModelProperty(value = "租户id") |
|||
private Integer tenantId; |
|||
|
|||
@ApiModelProperty(value = "创建时间") |
|||
private Date createTime; |
|||
|
|||
@ApiModelProperty(value = "修改时间") |
|||
private Date updateTime; |
|||
|
|||
} |
@ -0,0 +1,99 @@ |
|||
package com.gxwebsoft.tower.entity; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.IdType; |
|||
import com.baomidou.mybatisplus.annotation.TableField; |
|||
import com.baomidou.mybatisplus.annotation.TableId; |
|||
import java.time.LocalDateTime; |
|||
import com.baomidou.mybatisplus.annotation.TableLogic; |
|||
import java.io.Serializable; |
|||
import java.util.Date; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
import lombok.EqualsAndHashCode; |
|||
|
|||
/** |
|||
* 保养记录 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-22 13:44:23 |
|||
*/ |
|||
@Data |
|||
@EqualsAndHashCode(callSuper = false) |
|||
@ApiModel(value = "TowerSecurityRecord对象", description = "保养记录") |
|||
public class TowerSecurityRecord implements Serializable { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty(value = "自增ID") |
|||
@TableId(value = "security_record_id", type = IdType.AUTO) |
|||
private Integer securityRecordId; |
|||
|
|||
@ApiModelProperty(value = "保养计划ID") |
|||
private Integer securityId; |
|||
|
|||
@ApiModelProperty(value = "保养编号") |
|||
private String securityCode; |
|||
|
|||
@ApiModelProperty(value = "现场图片") |
|||
private String images; |
|||
|
|||
@ApiModelProperty(value = "经度") |
|||
private String longitude; |
|||
|
|||
@ApiModelProperty(value = "纬度") |
|||
private String latitude; |
|||
|
|||
@ApiModelProperty(value = "所在国家") |
|||
private String country; |
|||
|
|||
@ApiModelProperty(value = "所在省份") |
|||
private String province; |
|||
|
|||
@ApiModelProperty(value = "所在城市") |
|||
private String city; |
|||
|
|||
@ApiModelProperty(value = "所在辖区") |
|||
private String region; |
|||
|
|||
@ApiModelProperty(value = "区域名称") |
|||
private String regionName; |
|||
|
|||
@ApiModelProperty(value = "状态, 0正常, 1待修,2异常已修,3异常未修") |
|||
private Integer status; |
|||
|
|||
@ApiModelProperty(value = "备注") |
|||
private String comments; |
|||
|
|||
@ApiModelProperty(value = "排序号") |
|||
private Integer sortNumber; |
|||
|
|||
@ApiModelProperty(value = "所有人") |
|||
private Integer userId; |
|||
|
|||
@ApiModelProperty(value = "是否删除, 0否, 1是") |
|||
@TableLogic |
|||
private Integer deleted; |
|||
|
|||
@ApiModelProperty(value = "租户id") |
|||
private Integer tenantId; |
|||
|
|||
@ApiModelProperty(value = "创建时间") |
|||
private Date createTime; |
|||
|
|||
@ApiModelProperty(value = "修改时间") |
|||
private Date updateTime; |
|||
|
|||
@ApiModelProperty(value = "昵称") |
|||
@TableField(exist = false) |
|||
private String nickname; |
|||
|
|||
@ApiModelProperty(value = "手机号码") |
|||
@TableField(exist = false) |
|||
private String phone; |
|||
|
|||
@ApiModelProperty(value = "头像") |
|||
@TableField(exist = false) |
|||
private String avatar; |
|||
|
|||
} |
@ -0,0 +1,37 @@ |
|||
package com.gxwebsoft.tower.mapper; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.gxwebsoft.tower.entity.TowerSecurity; |
|||
import com.gxwebsoft.tower.param.TowerSecurityParam; |
|||
import org.apache.ibatis.annotations.Param; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 安全检查Mapper |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-22 13:44:23 |
|||
*/ |
|||
public interface TowerSecurityMapper extends BaseMapper<TowerSecurity> { |
|||
|
|||
/** |
|||
* 分页查询 |
|||
* |
|||
* @param page 分页对象 |
|||
* @param param 查询参数 |
|||
* @return List<TowerSecurity> |
|||
*/ |
|||
List<TowerSecurity> selectPageRel(@Param("page") IPage<TowerSecurity> page, |
|||
@Param("param") TowerSecurityParam param); |
|||
|
|||
/** |
|||
* 查询全部 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return List<User> |
|||
*/ |
|||
List<TowerSecurity> selectListRel(@Param("param") TowerSecurityParam param); |
|||
|
|||
} |
@ -0,0 +1,37 @@ |
|||
package com.gxwebsoft.tower.mapper; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.gxwebsoft.tower.entity.TowerSecurityRecord; |
|||
import com.gxwebsoft.tower.param.TowerSecurityRecordParam; |
|||
import org.apache.ibatis.annotations.Param; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 保养记录Mapper |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-22 13:44:23 |
|||
*/ |
|||
public interface TowerSecurityRecordMapper extends BaseMapper<TowerSecurityRecord> { |
|||
|
|||
/** |
|||
* 分页查询 |
|||
* |
|||
* @param page 分页对象 |
|||
* @param param 查询参数 |
|||
* @return List<TowerSecurityRecord> |
|||
*/ |
|||
List<TowerSecurityRecord> selectPageRel(@Param("page") IPage<TowerSecurityRecord> page, |
|||
@Param("param") TowerSecurityRecordParam param); |
|||
|
|||
/** |
|||
* 查询全部 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return List<User> |
|||
*/ |
|||
List<TowerSecurityRecord> selectListRel(@Param("param") TowerSecurityRecordParam param); |
|||
|
|||
} |
@ -0,0 +1,89 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.gxwebsoft.tower.mapper.TowerSecurityMapper"> |
|||
|
|||
<!-- 关联查询sql --> |
|||
<sql id="selectSql"> |
|||
SELECT a.* |
|||
FROM tower_security a |
|||
<where> |
|||
<if test="param.securityId != null"> |
|||
AND a.security_id = #{param.securityId} |
|||
</if> |
|||
<if test="param.type != null"> |
|||
AND a.type = #{param.type} |
|||
</if> |
|||
<if test="param.projectName != null"> |
|||
AND a.project_name LIKE CONCAT('%', #{param.projectName}, '%') |
|||
</if> |
|||
<if test="param.securityCode != null"> |
|||
AND a.security_code LIKE CONCAT('%', #{param.securityCode}, '%') |
|||
</if> |
|||
<if test="param.address != null"> |
|||
AND a.address LIKE CONCAT('%', #{param.address}, '%') |
|||
</if> |
|||
<if test="param.longitude != null"> |
|||
AND a.longitude LIKE CONCAT('%', #{param.longitude}, '%') |
|||
</if> |
|||
<if test="param.latitude != null"> |
|||
AND a.latitude LIKE CONCAT('%', #{param.latitude}, '%') |
|||
</if> |
|||
<if test="param.country != null"> |
|||
AND a.country LIKE CONCAT('%', #{param.country}, '%') |
|||
</if> |
|||
<if test="param.province != null"> |
|||
AND a.province LIKE CONCAT('%', #{param.province}, '%') |
|||
</if> |
|||
<if test="param.city != null"> |
|||
AND a.city LIKE CONCAT('%', #{param.city}, '%') |
|||
</if> |
|||
<if test="param.region != null"> |
|||
AND a.region LIKE CONCAT('%', #{param.region}, '%') |
|||
</if> |
|||
<if test="param.regionName != null"> |
|||
AND a.region_name LIKE CONCAT('%', #{param.regionName}, '%') |
|||
</if> |
|||
<if test="param.status != null"> |
|||
AND a.status = #{param.status} |
|||
</if> |
|||
<if test="param.comments != null"> |
|||
AND a.comments LIKE CONCAT('%', #{param.comments}, '%') |
|||
</if> |
|||
<if test="param.sortNumber != null"> |
|||
AND a.sort_number = #{param.sortNumber} |
|||
</if> |
|||
<if test="param.userId != null"> |
|||
AND a.user_id = #{param.userId} |
|||
</if> |
|||
<if test="param.deleted != null"> |
|||
AND a.deleted = #{param.deleted} |
|||
</if> |
|||
<if test="param.deleted == null"> |
|||
AND a.deleted = 0 |
|||
</if> |
|||
<if test="param.createTimeStart != null"> |
|||
AND a.create_time >= #{param.createTimeStart} |
|||
</if> |
|||
<if test="param.createTimeEnd != null"> |
|||
AND a.create_time <= #{param.createTimeEnd} |
|||
</if> |
|||
<if test="param.keywords != null"> |
|||
AND (a.project_name LIKE CONCAT('%', #{param.keywords}, '%') |
|||
OR a.project_id = #{param.keywords} |
|||
OR a.security_code = #{param.keywords} |
|||
) |
|||
</if> |
|||
</where> |
|||
</sql> |
|||
|
|||
<!-- 分页查询 --> |
|||
<select id="selectPageRel" resultType="com.gxwebsoft.tower.entity.TowerSecurity"> |
|||
<include refid="selectSql"></include> |
|||
</select> |
|||
|
|||
<!-- 查询全部 --> |
|||
<select id="selectListRel" resultType="com.gxwebsoft.tower.entity.TowerSecurity"> |
|||
<include refid="selectSql"></include> |
|||
</select> |
|||
|
|||
</mapper> |
@ -0,0 +1,78 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.gxwebsoft.tower.mapper.TowerSecurityRecordMapper"> |
|||
|
|||
<!-- 关联查询sql --> |
|||
<sql id="selectSql"> |
|||
SELECT a.*,b.nickname,b.phone,b.avatar |
|||
FROM tower_security_record a |
|||
LEFT JOIN sys_user b ON a.user_id = b.user_id |
|||
<where> |
|||
<if test="param.securityId != null"> |
|||
AND a.security_id = #{param.securityId} |
|||
</if> |
|||
<if test="param.securityCode != null"> |
|||
AND a.security_code LIKE CONCAT('%', #{param.securityCode}, '%') |
|||
</if> |
|||
<if test="param.images != null"> |
|||
AND a.images LIKE CONCAT('%', #{param.images}, '%') |
|||
</if> |
|||
<if test="param.longitude != null"> |
|||
AND a.longitude LIKE CONCAT('%', #{param.longitude}, '%') |
|||
</if> |
|||
<if test="param.latitude != null"> |
|||
AND a.latitude LIKE CONCAT('%', #{param.latitude}, '%') |
|||
</if> |
|||
<if test="param.country != null"> |
|||
AND a.country LIKE CONCAT('%', #{param.country}, '%') |
|||
</if> |
|||
<if test="param.province != null"> |
|||
AND a.province LIKE CONCAT('%', #{param.province}, '%') |
|||
</if> |
|||
<if test="param.city != null"> |
|||
AND a.city LIKE CONCAT('%', #{param.city}, '%') |
|||
</if> |
|||
<if test="param.region != null"> |
|||
AND a.region LIKE CONCAT('%', #{param.region}, '%') |
|||
</if> |
|||
<if test="param.regionName != null"> |
|||
AND a.region_name LIKE CONCAT('%', #{param.regionName}, '%') |
|||
</if> |
|||
<if test="param.status != null"> |
|||
AND a.status = #{param.status} |
|||
</if> |
|||
<if test="param.comments != null"> |
|||
AND a.comments LIKE CONCAT('%', #{param.comments}, '%') |
|||
</if> |
|||
<if test="param.sortNumber != null"> |
|||
AND a.sort_number = #{param.sortNumber} |
|||
</if> |
|||
<if test="param.userId != null"> |
|||
AND a.user_id = #{param.userId} |
|||
</if> |
|||
<if test="param.deleted != null"> |
|||
AND a.deleted = #{param.deleted} |
|||
</if> |
|||
<if test="param.deleted == null"> |
|||
AND a.deleted = 0 |
|||
</if> |
|||
<if test="param.createTimeStart != null"> |
|||
AND a.create_time >= #{param.createTimeStart} |
|||
</if> |
|||
<if test="param.createTimeEnd != null"> |
|||
AND a.create_time <= #{param.createTimeEnd} |
|||
</if> |
|||
</where> |
|||
</sql> |
|||
|
|||
<!-- 分页查询 --> |
|||
<select id="selectPageRel" resultType="com.gxwebsoft.tower.entity.TowerSecurityRecord"> |
|||
<include refid="selectSql"></include> |
|||
</select> |
|||
|
|||
<!-- 查询全部 --> |
|||
<select id="selectListRel" resultType="com.gxwebsoft.tower.entity.TowerSecurityRecord"> |
|||
<include refid="selectSql"></include> |
|||
</select> |
|||
|
|||
</mapper> |
@ -0,0 +1,81 @@ |
|||
package com.gxwebsoft.tower.param; |
|||
|
|||
import com.gxwebsoft.common.core.annotation.QueryField; |
|||
import com.gxwebsoft.common.core.annotation.QueryType; |
|||
import com.gxwebsoft.common.core.web.BaseParam; |
|||
import com.fasterxml.jackson.annotation.JsonInclude; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
import lombok.EqualsAndHashCode; |
|||
|
|||
/** |
|||
* 安全检查查询参数 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-22 13:44:23 |
|||
*/ |
|||
@Data |
|||
@EqualsAndHashCode(callSuper = false) |
|||
@JsonInclude(JsonInclude.Include.NON_NULL) |
|||
@ApiModel(value = "TowerSecurityParam对象", description = "安全检查查询参数") |
|||
public class TowerSecurityParam extends BaseParam { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty(value = "自增ID") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer securityId; |
|||
|
|||
@ApiModelProperty(value = "类型") |
|||
private Integer type; |
|||
|
|||
@ApiModelProperty(value = "项目名称") |
|||
private String projectName; |
|||
|
|||
@ApiModelProperty(value = "保养编号") |
|||
private String securityCode; |
|||
|
|||
@ApiModelProperty(value = "所在地") |
|||
private String address; |
|||
|
|||
@ApiModelProperty(value = "经度") |
|||
private String longitude; |
|||
|
|||
@ApiModelProperty(value = "纬度") |
|||
private String latitude; |
|||
|
|||
@ApiModelProperty(value = "所在国家") |
|||
private String country; |
|||
|
|||
@ApiModelProperty(value = "所在省份") |
|||
private String province; |
|||
|
|||
@ApiModelProperty(value = "所在城市") |
|||
private String city; |
|||
|
|||
@ApiModelProperty(value = "所在辖区") |
|||
private String region; |
|||
|
|||
@ApiModelProperty(value = "区域名称") |
|||
private String regionName; |
|||
|
|||
@ApiModelProperty(value = "状态, 0正常, 1待修,2异常已修,3异常未修") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer status; |
|||
|
|||
@ApiModelProperty(value = "备注") |
|||
private String comments; |
|||
|
|||
@ApiModelProperty(value = "排序号") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer sortNumber; |
|||
|
|||
@ApiModelProperty(value = "所有人") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer userId; |
|||
|
|||
@ApiModelProperty(value = "是否删除, 0否, 1是") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer deleted; |
|||
|
|||
} |
@ -0,0 +1,75 @@ |
|||
package com.gxwebsoft.tower.param; |
|||
|
|||
import com.gxwebsoft.common.core.annotation.QueryField; |
|||
import com.gxwebsoft.common.core.annotation.QueryType; |
|||
import com.gxwebsoft.common.core.web.BaseParam; |
|||
import com.fasterxml.jackson.annotation.JsonInclude; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
import lombok.EqualsAndHashCode; |
|||
|
|||
/** |
|||
* 保养记录查询参数 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-22 13:44:23 |
|||
*/ |
|||
@Data |
|||
@EqualsAndHashCode(callSuper = false) |
|||
@JsonInclude(JsonInclude.Include.NON_NULL) |
|||
@ApiModel(value = "TowerSecurityRecordParam对象", description = "保养记录查询参数") |
|||
public class TowerSecurityRecordParam extends BaseParam { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty(value = "自增ID") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer securityId; |
|||
|
|||
@ApiModelProperty(value = "保养编号") |
|||
private String securityCode; |
|||
|
|||
@ApiModelProperty(value = "现场图片") |
|||
private String images; |
|||
|
|||
@ApiModelProperty(value = "经度") |
|||
private String longitude; |
|||
|
|||
@ApiModelProperty(value = "纬度") |
|||
private String latitude; |
|||
|
|||
@ApiModelProperty(value = "所在国家") |
|||
private String country; |
|||
|
|||
@ApiModelProperty(value = "所在省份") |
|||
private String province; |
|||
|
|||
@ApiModelProperty(value = "所在城市") |
|||
private String city; |
|||
|
|||
@ApiModelProperty(value = "所在辖区") |
|||
private String region; |
|||
|
|||
@ApiModelProperty(value = "区域名称") |
|||
private String regionName; |
|||
|
|||
@ApiModelProperty(value = "状态, 0正常, 1待修,2异常已修,3异常未修") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer status; |
|||
|
|||
@ApiModelProperty(value = "备注") |
|||
private String comments; |
|||
|
|||
@ApiModelProperty(value = "排序号") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer sortNumber; |
|||
|
|||
@ApiModelProperty(value = "所有人") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer userId; |
|||
|
|||
@ApiModelProperty(value = "是否删除, 0否, 1是") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer deleted; |
|||
|
|||
} |
@ -0,0 +1,42 @@ |
|||
package com.gxwebsoft.tower.service; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.IService; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import com.gxwebsoft.tower.entity.TowerSecurityRecord; |
|||
import com.gxwebsoft.tower.param.TowerSecurityRecordParam; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 保养记录Service |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-22 13:44:23 |
|||
*/ |
|||
public interface TowerSecurityRecordService extends IService<TowerSecurityRecord> { |
|||
|
|||
/** |
|||
* 分页关联查询 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return PageResult<TowerSecurityRecord> |
|||
*/ |
|||
PageResult<TowerSecurityRecord> pageRel(TowerSecurityRecordParam param); |
|||
|
|||
/** |
|||
* 关联查询全部 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return List<TowerSecurityRecord> |
|||
*/ |
|||
List<TowerSecurityRecord> listRel(TowerSecurityRecordParam param); |
|||
|
|||
/** |
|||
* 根据id查询 |
|||
* |
|||
* @param securityId 自增ID |
|||
* @return TowerSecurityRecord |
|||
*/ |
|||
TowerSecurityRecord getByIdRel(Integer securityId); |
|||
|
|||
} |
@ -0,0 +1,42 @@ |
|||
package com.gxwebsoft.tower.service; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.IService; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import com.gxwebsoft.tower.entity.TowerSecurity; |
|||
import com.gxwebsoft.tower.param.TowerSecurityParam; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 安全检查Service |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-22 13:44:23 |
|||
*/ |
|||
public interface TowerSecurityService extends IService<TowerSecurity> { |
|||
|
|||
/** |
|||
* 分页关联查询 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return PageResult<TowerSecurity> |
|||
*/ |
|||
PageResult<TowerSecurity> pageRel(TowerSecurityParam param); |
|||
|
|||
/** |
|||
* 关联查询全部 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return List<TowerSecurity> |
|||
*/ |
|||
List<TowerSecurity> listRel(TowerSecurityParam param); |
|||
|
|||
/** |
|||
* 根据id查询 |
|||
* |
|||
* @param securityId 自增ID |
|||
* @return TowerSecurity |
|||
*/ |
|||
TowerSecurity getByIdRel(Integer securityId); |
|||
|
|||
} |
@ -0,0 +1,47 @@ |
|||
package com.gxwebsoft.tower.service.impl; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; |
|||
import com.gxwebsoft.tower.mapper.TowerSecurityRecordMapper; |
|||
import com.gxwebsoft.tower.service.TowerSecurityRecordService; |
|||
import com.gxwebsoft.tower.entity.TowerSecurityRecord; |
|||
import com.gxwebsoft.tower.param.TowerSecurityRecordParam; |
|||
import com.gxwebsoft.common.core.web.PageParam; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 保养记录Service实现 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-22 13:44:23 |
|||
*/ |
|||
@Service |
|||
public class TowerSecurityRecordServiceImpl extends ServiceImpl<TowerSecurityRecordMapper, TowerSecurityRecord> implements TowerSecurityRecordService { |
|||
|
|||
@Override |
|||
public PageResult<TowerSecurityRecord> pageRel(TowerSecurityRecordParam param) { |
|||
PageParam<TowerSecurityRecord, TowerSecurityRecordParam> page = new PageParam<>(param); |
|||
//page.setDefaultOrder("create_time desc");
|
|||
List<TowerSecurityRecord> list = baseMapper.selectPageRel(page, param); |
|||
return new PageResult<>(list, page.getTotal()); |
|||
} |
|||
|
|||
@Override |
|||
public List<TowerSecurityRecord> listRel(TowerSecurityRecordParam param) { |
|||
List<TowerSecurityRecord> list = baseMapper.selectListRel(param); |
|||
// 排序
|
|||
PageParam<TowerSecurityRecord, TowerSecurityRecordParam> page = new PageParam<>(); |
|||
//page.setDefaultOrder("create_time desc");
|
|||
return page.sortRecords(list); |
|||
} |
|||
|
|||
@Override |
|||
public TowerSecurityRecord getByIdRel(Integer securityId) { |
|||
TowerSecurityRecordParam param = new TowerSecurityRecordParam(); |
|||
param.setSecurityId(securityId); |
|||
return param.getOne(baseMapper.selectListRel(param)); |
|||
} |
|||
|
|||
} |
@ -0,0 +1,47 @@ |
|||
package com.gxwebsoft.tower.service.impl; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; |
|||
import com.gxwebsoft.tower.mapper.TowerSecurityMapper; |
|||
import com.gxwebsoft.tower.service.TowerSecurityService; |
|||
import com.gxwebsoft.tower.entity.TowerSecurity; |
|||
import com.gxwebsoft.tower.param.TowerSecurityParam; |
|||
import com.gxwebsoft.common.core.web.PageParam; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 安全检查Service实现 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-22 13:44:23 |
|||
*/ |
|||
@Service |
|||
public class TowerSecurityServiceImpl extends ServiceImpl<TowerSecurityMapper, TowerSecurity> implements TowerSecurityService { |
|||
|
|||
@Override |
|||
public PageResult<TowerSecurity> pageRel(TowerSecurityParam param) { |
|||
PageParam<TowerSecurity, TowerSecurityParam> page = new PageParam<>(param); |
|||
page.setDefaultOrder("create_time desc"); |
|||
List<TowerSecurity> list = baseMapper.selectPageRel(page, param); |
|||
return new PageResult<>(list, page.getTotal()); |
|||
} |
|||
|
|||
@Override |
|||
public List<TowerSecurity> listRel(TowerSecurityParam param) { |
|||
List<TowerSecurity> list = baseMapper.selectListRel(param); |
|||
// 排序
|
|||
PageParam<TowerSecurity, TowerSecurityParam> page = new PageParam<>(); |
|||
//page.setDefaultOrder("create_time desc");
|
|||
return page.sortRecords(list); |
|||
} |
|||
|
|||
@Override |
|||
public TowerSecurity getByIdRel(Integer securityId) { |
|||
TowerSecurityParam param = new TowerSecurityParam(); |
|||
param.setSecurityId(securityId); |
|||
return param.getOne(baseMapper.selectListRel(param)); |
|||
} |
|||
|
|||
} |
Loading…
Reference in new issue