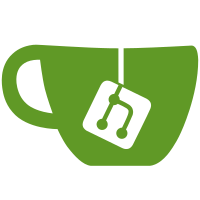
10 changed files with 6686 additions and 2 deletions
@ -0,0 +1,136 @@ |
|||
package com.gxwebsoft.tower.controller; |
|||
|
|||
import com.gxwebsoft.common.core.web.BaseController; |
|||
import com.gxwebsoft.common.system.entity.User; |
|||
import com.gxwebsoft.tower.service.TowerRentService; |
|||
import com.gxwebsoft.tower.entity.TowerRent; |
|||
import com.gxwebsoft.tower.param.TowerRentParam; |
|||
import com.gxwebsoft.common.core.web.ApiResult; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import com.gxwebsoft.common.core.web.PageParam; |
|||
import com.gxwebsoft.common.core.web.BatchParam; |
|||
import com.gxwebsoft.common.core.annotation.OperationLog; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.security.access.prepost.PreAuthorize; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import javax.annotation.Resource; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 标准节租金控制器 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-05 15:04:19 |
|||
*/ |
|||
@Api(tags = "标准节租金管理") |
|||
@RestController |
|||
@RequestMapping("/api/tower/tower-rent") |
|||
public class TowerRentController extends BaseController { |
|||
@Resource |
|||
private TowerRentService towerRentService; |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerRent:list')") |
|||
@OperationLog |
|||
@ApiOperation("分页查询标准节租金") |
|||
@GetMapping("/page") |
|||
public ApiResult<PageResult<TowerRent>> page(TowerRentParam param) { |
|||
// 使用关联查询
|
|||
return success(towerRentService.pageRel(param)); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerRent:list')") |
|||
@OperationLog |
|||
@ApiOperation("查询全部标准节租金") |
|||
@GetMapping() |
|||
public ApiResult<List<TowerRent>> list(TowerRentParam param) { |
|||
PageParam<TowerRent, TowerRentParam> page = new PageParam<>(param); |
|||
page.setDefaultOrder("create_time desc"); |
|||
return success(towerRentService.list(page.getOrderWrapper())); |
|||
// 使用关联查询
|
|||
//return success(towerRentService.listRel(param));
|
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerRent:list')") |
|||
@OperationLog |
|||
@ApiOperation("根据id查询标准节租金") |
|||
@GetMapping("/{id}") |
|||
public ApiResult<TowerRent> get(@PathVariable("id") Integer id) { |
|||
return success(towerRentService.getById(id)); |
|||
// 使用关联查询
|
|||
//return success(towerRentService.getByIdRel(id));
|
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerRent:save')") |
|||
@OperationLog |
|||
@ApiOperation("添加标准节租金") |
|||
@PostMapping() |
|||
public ApiResult<?> save(@RequestBody TowerRent towerRent) { |
|||
// 记录当前登录用户id
|
|||
User loginUser = getLoginUser(); |
|||
if (loginUser != null) { |
|||
towerRent.setUserId(loginUser.getUserId()); |
|||
} |
|||
if (towerRentService.save(towerRent)) { |
|||
return success("添加成功"); |
|||
} |
|||
return fail("添加失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerRent:update')") |
|||
@OperationLog |
|||
@ApiOperation("修改标准节租金") |
|||
@PutMapping() |
|||
public ApiResult<?> update(@RequestBody TowerRent towerRent) { |
|||
if (towerRentService.updateById(towerRent)) { |
|||
return success("修改成功"); |
|||
} |
|||
return fail("修改失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerRent:remove')") |
|||
@OperationLog |
|||
@ApiOperation("删除标准节租金") |
|||
@DeleteMapping("/{id}") |
|||
public ApiResult<?> remove(@PathVariable("id") Integer id) { |
|||
if (towerRentService.removeById(id)) { |
|||
return success("删除成功"); |
|||
} |
|||
return fail("删除失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerRent:save')") |
|||
@OperationLog |
|||
@ApiOperation("批量添加标准节租金") |
|||
@PostMapping("/batch") |
|||
public ApiResult<?> saveBatch(@RequestBody List<TowerRent> list) { |
|||
if (towerRentService.saveBatch(list)) { |
|||
return success("添加成功"); |
|||
} |
|||
return fail("添加失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerRent:update')") |
|||
@OperationLog |
|||
@ApiOperation("批量修改标准节租金") |
|||
@PutMapping("/batch") |
|||
public ApiResult<?> removeBatch(@RequestBody BatchParam<TowerRent> batchParam) { |
|||
if (batchParam.update(towerRentService, "rent_id")) { |
|||
return success("修改成功"); |
|||
} |
|||
return fail("修改失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerRent:remove')") |
|||
@OperationLog |
|||
@ApiOperation("批量删除标准节租金") |
|||
@DeleteMapping("/batch") |
|||
public ApiResult<?> removeBatch(@RequestBody List<Integer> ids) { |
|||
if (towerRentService.removeByIds(ids)) { |
|||
return success("删除成功"); |
|||
} |
|||
return fail("删除失败"); |
|||
} |
|||
|
|||
} |
@ -0,0 +1,103 @@ |
|||
package com.gxwebsoft.tower.entity; |
|||
|
|||
import java.math.BigDecimal; |
|||
import com.baomidou.mybatisplus.annotation.IdType; |
|||
import com.baomidou.mybatisplus.annotation.TableField; |
|||
import com.baomidou.mybatisplus.annotation.TableId; |
|||
import java.time.LocalDateTime; |
|||
import com.baomidou.mybatisplus.annotation.TableLogic; |
|||
import java.io.Serializable; |
|||
import java.util.Date; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
import lombok.EqualsAndHashCode; |
|||
|
|||
/** |
|||
* 标准节租金 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-05 15:04:19 |
|||
*/ |
|||
@Data |
|||
@EqualsAndHashCode(callSuper = false) |
|||
@ApiModel(value = "TowerRent对象", description = "标准节租金") |
|||
public class TowerRent implements Serializable { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty(value = "租单记录id") |
|||
@TableId(value = "rent_id", type = IdType.AUTO) |
|||
private Integer rentId; |
|||
|
|||
@ApiModelProperty(value = "名称") |
|||
private String name; |
|||
|
|||
@ApiModelProperty(value = "租金") |
|||
private BigDecimal rent; |
|||
|
|||
@ApiModelProperty(value = "每个月租金") |
|||
private BigDecimal month; |
|||
|
|||
@ApiModelProperty(value = "月数") |
|||
private Integer num; |
|||
|
|||
@ApiModelProperty(value = "获取的租金") |
|||
private BigDecimal totalRent; |
|||
|
|||
@ApiModelProperty(value = "项目ID") |
|||
private Integer projectId; |
|||
|
|||
@ApiModelProperty(value = "合同编号") |
|||
private String contractNo; |
|||
|
|||
@ApiModelProperty(value = "设备ID") |
|||
private Integer equipmentId; |
|||
|
|||
@ApiModelProperty(value = "起租日期") |
|||
private String startDate; |
|||
|
|||
@ApiModelProperty(value = "停租日期") |
|||
private String stopDate; |
|||
|
|||
@ApiModelProperty(value = "检测日期") |
|||
private String checkDate; |
|||
|
|||
@ApiModelProperty(value = "报停日期") |
|||
private String reportStopDate; |
|||
|
|||
@ApiModelProperty(value = "报停类型") |
|||
private String reportStopType; |
|||
|
|||
@ApiModelProperty(value = "复工日期") |
|||
private String restoreDate; |
|||
|
|||
@ApiModelProperty(value = "报监编号") |
|||
private String reportMonitorNo; |
|||
|
|||
@ApiModelProperty(value = "承租单位") |
|||
private Integer companyId; |
|||
|
|||
@ApiModelProperty(value = "企业名称") |
|||
private String companyName; |
|||
|
|||
@ApiModelProperty(value = "状态(0未起租1起租2中途报停3报停复工4拆卸停租)") |
|||
private Boolean status; |
|||
|
|||
@ApiModelProperty(value = "所有人") |
|||
private Integer userId; |
|||
|
|||
@ApiModelProperty(value = "是否删除, 0否, 1是") |
|||
@TableLogic |
|||
private Integer deleted; |
|||
|
|||
@ApiModelProperty(value = "租户id") |
|||
private Integer tenantId; |
|||
|
|||
@ApiModelProperty(value = "创建时间") |
|||
private Date createTime; |
|||
|
|||
@ApiModelProperty(value = "修改时间") |
|||
private Date updateTime; |
|||
|
|||
} |
@ -0,0 +1,37 @@ |
|||
package com.gxwebsoft.tower.mapper; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.gxwebsoft.tower.entity.TowerRent; |
|||
import com.gxwebsoft.tower.param.TowerRentParam; |
|||
import org.apache.ibatis.annotations.Param; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 标准节租金Mapper |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-05 15:04:19 |
|||
*/ |
|||
public interface TowerRentMapper extends BaseMapper<TowerRent> { |
|||
|
|||
/** |
|||
* 分页查询 |
|||
* |
|||
* @param page 分页对象 |
|||
* @param param 查询参数 |
|||
* @return List<TowerRent> |
|||
*/ |
|||
List<TowerRent> selectPageRel(@Param("page") IPage<TowerRent> page, |
|||
@Param("param") TowerRentParam param); |
|||
|
|||
/** |
|||
* 查询全部 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return List<User> |
|||
*/ |
|||
List<TowerRent> selectListRel(@Param("param") TowerRentParam param); |
|||
|
|||
} |
@ -0,0 +1,87 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.gxwebsoft.tower.mapper.TowerRentMapper"> |
|||
|
|||
<!-- 关联查询sql --> |
|||
<sql id="selectSql"> |
|||
SELECT a.*, b.company_name |
|||
FROM tower_rent a |
|||
LEFT JOIN sys_company b ON a.company_id = b.company_id |
|||
<where> |
|||
<if test="param.rentId != null"> |
|||
AND a.rent_id = #{param.rentId} |
|||
</if> |
|||
<if test="param.rent != null"> |
|||
AND a.rent = #{param.rent} |
|||
</if> |
|||
<if test="param.month != null"> |
|||
AND a.month = #{param.month} |
|||
</if> |
|||
<if test="param.totalRent != null"> |
|||
AND a.total_rent = #{param.totalRent} |
|||
</if> |
|||
<if test="param.projectId != null"> |
|||
AND a.project_id = #{param.projectId} |
|||
</if> |
|||
<if test="param.equipmentId != null"> |
|||
AND a.equipment_id = #{param.equipmentId} |
|||
</if> |
|||
<if test="param.startDate != null"> |
|||
AND a.start_date LIKE CONCAT('%', #{param.startDate}, '%') |
|||
</if> |
|||
<if test="param.stopDate != null"> |
|||
AND a.stop_date LIKE CONCAT('%', #{param.stopDate}, '%') |
|||
</if> |
|||
<if test="param.checkDate != null"> |
|||
AND a.check_date LIKE CONCAT('%', #{param.checkDate}, '%') |
|||
</if> |
|||
<if test="param.reportStopDate != null"> |
|||
AND a.report_stop_date LIKE CONCAT('%', #{param.reportStopDate}, '%') |
|||
</if> |
|||
<if test="param.reportStopType != null"> |
|||
AND a.report_stop_type LIKE CONCAT('%', #{param.reportStopType}, '%') |
|||
</if> |
|||
<if test="param.restoreDate != null"> |
|||
AND a.restore_date LIKE CONCAT('%', #{param.restoreDate}, '%') |
|||
</if> |
|||
<if test="param.reportMonitorNo != null"> |
|||
AND a.report_monitor_no LIKE CONCAT('%', #{param.reportMonitorNo}, '%') |
|||
</if> |
|||
<if test="param.companyId != null"> |
|||
AND a.company_id = #{param.companyId} |
|||
</if> |
|||
<if test="param.status != null"> |
|||
AND a.status = #{param.status} |
|||
</if> |
|||
<if test="param.userId != null"> |
|||
AND a.user_id = #{param.userId} |
|||
</if> |
|||
<if test="param.deleted != null"> |
|||
AND a.deleted = #{param.deleted} |
|||
</if> |
|||
<if test="param.deleted == null"> |
|||
AND a.deleted = 0 |
|||
</if> |
|||
<if test="param.createTimeStart != null"> |
|||
AND a.create_time >= #{param.createTimeStart} |
|||
</if> |
|||
<if test="param.createTimeEnd != null"> |
|||
AND a.create_time <= #{param.createTimeEnd} |
|||
</if> |
|||
<if test="param.keywords != null"> |
|||
AND ( a.company_name LIKE CONCAT('%', #{param.keywords}, '%') ) |
|||
</if> |
|||
</where> |
|||
</sql> |
|||
|
|||
<!-- 分页查询 --> |
|||
<select id="selectPageRel" resultType="com.gxwebsoft.tower.entity.TowerRent"> |
|||
<include refid="selectSql"></include> |
|||
</select> |
|||
|
|||
<!-- 查询全部 --> |
|||
<select id="selectListRel" resultType="com.gxwebsoft.tower.entity.TowerRent"> |
|||
<include refid="selectSql"></include> |
|||
</select> |
|||
|
|||
</mapper> |
@ -0,0 +1,100 @@ |
|||
package com.gxwebsoft.tower.param; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableField; |
|||
import com.gxwebsoft.common.core.annotation.QueryField; |
|||
import com.gxwebsoft.common.core.annotation.QueryType; |
|||
import com.gxwebsoft.common.core.web.BaseParam; |
|||
import com.fasterxml.jackson.annotation.JsonInclude; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
import lombok.EqualsAndHashCode; |
|||
|
|||
import java.math.BigDecimal; |
|||
|
|||
/** |
|||
* 标准节租金查询参数 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-05 15:04:19 |
|||
*/ |
|||
@Data |
|||
@EqualsAndHashCode(callSuper = false) |
|||
@JsonInclude(JsonInclude.Include.NON_NULL) |
|||
@ApiModel(value = "TowerRentParam对象", description = "标准节租金查询参数") |
|||
public class TowerRentParam extends BaseParam { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty(value = "租单记录id") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer rentId; |
|||
|
|||
@ApiModelProperty(value = "租金") |
|||
@QueryField(type = QueryType.EQ) |
|||
private BigDecimal rent; |
|||
|
|||
@ApiModelProperty(value = "每个月租金") |
|||
@QueryField(type = QueryType.EQ) |
|||
private BigDecimal month; |
|||
|
|||
@ApiModelProperty(value = "月数") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer num; |
|||
|
|||
@ApiModelProperty(value = "获取的租金") |
|||
@QueryField(type = QueryType.EQ) |
|||
private BigDecimal totalRent; |
|||
|
|||
@ApiModelProperty(value = "项目ID") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer projectId; |
|||
|
|||
@ApiModelProperty(value = "合同编号") |
|||
private String contractNo; |
|||
|
|||
@ApiModelProperty(value = "设备ID") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer equipmentId; |
|||
|
|||
@ApiModelProperty(value = "起租日期") |
|||
private String startDate; |
|||
|
|||
@ApiModelProperty(value = "停租日期") |
|||
private String stopDate; |
|||
|
|||
@ApiModelProperty(value = "检测日期") |
|||
private String checkDate; |
|||
|
|||
@ApiModelProperty(value = "报停日期") |
|||
private String reportStopDate; |
|||
|
|||
@ApiModelProperty(value = "报停类型") |
|||
private String reportStopType; |
|||
|
|||
@ApiModelProperty(value = "复工日期") |
|||
private String restoreDate; |
|||
|
|||
@ApiModelProperty(value = "报监编号") |
|||
private String reportMonitorNo; |
|||
|
|||
@ApiModelProperty(value = "承租单位") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer companyId; |
|||
|
|||
@ApiModelProperty(value = "企业名称") |
|||
@TableField(exist = false) |
|||
private String companyName; |
|||
|
|||
@ApiModelProperty(value = "状态(0未起租1起租2中途报停3报停复工4拆卸停租)") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Boolean status; |
|||
|
|||
@ApiModelProperty(value = "所有人") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer userId; |
|||
|
|||
@ApiModelProperty(value = "是否删除, 0否, 1是") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer deleted; |
|||
|
|||
} |
@ -0,0 +1,42 @@ |
|||
package com.gxwebsoft.tower.service; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.IService; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import com.gxwebsoft.tower.entity.TowerRent; |
|||
import com.gxwebsoft.tower.param.TowerRentParam; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 标准节租金Service |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-05 15:04:19 |
|||
*/ |
|||
public interface TowerRentService extends IService<TowerRent> { |
|||
|
|||
/** |
|||
* 分页关联查询 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return PageResult<TowerRent> |
|||
*/ |
|||
PageResult<TowerRent> pageRel(TowerRentParam param); |
|||
|
|||
/** |
|||
* 关联查询全部 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return List<TowerRent> |
|||
*/ |
|||
List<TowerRent> listRel(TowerRentParam param); |
|||
|
|||
/** |
|||
* 根据id查询 |
|||
* |
|||
* @param rentId 租单记录id |
|||
* @return TowerRent |
|||
*/ |
|||
TowerRent getByIdRel(Integer rentId); |
|||
|
|||
} |
@ -0,0 +1,47 @@ |
|||
package com.gxwebsoft.tower.service.impl; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; |
|||
import com.gxwebsoft.tower.mapper.TowerRentMapper; |
|||
import com.gxwebsoft.tower.service.TowerRentService; |
|||
import com.gxwebsoft.tower.entity.TowerRent; |
|||
import com.gxwebsoft.tower.param.TowerRentParam; |
|||
import com.gxwebsoft.common.core.web.PageParam; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 标准节租金Service实现 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-05 15:04:19 |
|||
*/ |
|||
@Service |
|||
public class TowerRentServiceImpl extends ServiceImpl<TowerRentMapper, TowerRent> implements TowerRentService { |
|||
|
|||
@Override |
|||
public PageResult<TowerRent> pageRel(TowerRentParam param) { |
|||
PageParam<TowerRent, TowerRentParam> page = new PageParam<>(param); |
|||
//page.setDefaultOrder("create_time desc");
|
|||
List<TowerRent> list = baseMapper.selectPageRel(page, param); |
|||
return new PageResult<>(list, page.getTotal()); |
|||
} |
|||
|
|||
@Override |
|||
public List<TowerRent> listRel(TowerRentParam param) { |
|||
List<TowerRent> list = baseMapper.selectListRel(param); |
|||
// 排序
|
|||
PageParam<TowerRent, TowerRentParam> page = new PageParam<>(); |
|||
//page.setDefaultOrder("create_time desc");
|
|||
return page.sortRecords(list); |
|||
} |
|||
|
|||
@Override |
|||
public TowerRent getByIdRel(Integer rentId) { |
|||
TowerRentParam param = new TowerRentParam(); |
|||
param.setRentId(rentId); |
|||
return param.getOne(baseMapper.selectListRel(param)); |
|||
} |
|||
|
|||
} |
File diff suppressed because it is too large
Loading…
Reference in new issue