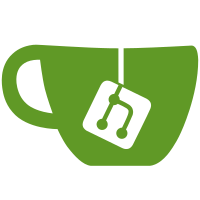
1 changed files with 0 additions and 295 deletions
@ -1,295 +0,0 @@ |
|||||
package com.gxwebsoft.open.controller; |
|
||||
|
|
||||
import cn.hutool.core.date.DateUtil; |
|
||||
import cn.hutool.core.util.StrUtil; |
|
||||
import cn.hutool.http.HttpUtil; |
|
||||
import com.alibaba.fastjson.JSONObject; |
|
||||
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
|
||||
import com.gxwebsoft.apps.entity.BcEquipment; |
|
||||
import com.gxwebsoft.apps.param.BcEquipmentParam; |
|
||||
import com.gxwebsoft.apps.service.BcEquipmentService; |
|
||||
import com.gxwebsoft.apps.utils.BcUtil; |
|
||||
import com.gxwebsoft.common.core.web.ApiResult; |
|
||||
import com.gxwebsoft.common.core.web.BaseController; |
|
||||
import com.gxwebsoft.common.system.entity.User; |
|
||||
import com.gxwebsoft.common.system.service.UserService; |
|
||||
import com.gxwebsoft.shop.entity.Order; |
|
||||
import com.gxwebsoft.shop.entity.OrderGoods; |
|
||||
import com.gxwebsoft.shop.entity.UserReferee; |
|
||||
import com.gxwebsoft.shop.param.OrderGoodsParam; |
|
||||
import com.gxwebsoft.shop.param.OrderParam; |
|
||||
import com.gxwebsoft.shop.param.UserRefereeParam; |
|
||||
import com.gxwebsoft.shop.service.OrderGoodsService; |
|
||||
import com.gxwebsoft.shop.service.OrderService; |
|
||||
import com.gxwebsoft.shop.service.UserRefereeService; |
|
||||
import io.swagger.annotations.Api; |
|
||||
import io.swagger.annotations.ApiOperation; |
|
||||
import org.springframework.transaction.annotation.Transactional; |
|
||||
import org.springframework.web.bind.annotation.PostMapping; |
|
||||
import org.springframework.web.bind.annotation.RequestBody; |
|
||||
import org.springframework.web.bind.annotation.RequestMapping; |
|
||||
import org.springframework.web.bind.annotation.RestController; |
|
||||
|
|
||||
import javax.annotation.Resource; |
|
||||
import javax.servlet.http.HttpServletRequest; |
|
||||
import java.util.HashMap; |
|
||||
import java.util.List; |
|
||||
import java.util.stream.Collectors; |
|
||||
|
|
||||
import static com.gxwebsoft.common.core.constants.OrderConstants.*; |
|
||||
import static com.gxwebsoft.shop.constants.OrderConstants.ORDER_GOODS_DELIVERY_STATUS20; |
|
||||
|
|
||||
/** |
|
||||
* 云报餐机器接口 |
|
||||
* |
|
||||
* @author 科技小王子 |
|
||||
* @since 2023-04-24 19:25:59 |
|
||||
*/ |
|
||||
@Api(tags = "云报餐机器接口") |
|
||||
@RestController |
|
||||
@RequestMapping("/hxz/v1") |
|
||||
public class OpenBcController extends BaseController { |
|
||||
@Resource |
|
||||
private BcUtil bcUtil; |
|
||||
@Resource |
|
||||
private OrderService orderService; |
|
||||
@Resource |
|
||||
private UserService userService; |
|
||||
@Resource |
|
||||
private OrderGoodsService orderGoodsService; |
|
||||
@Resource |
|
||||
private UserRefereeService userRefereeService; |
|
||||
@Resource |
|
||||
private BcEquipmentService bcEquipmentService; |
|
||||
|
|
||||
@ApiOperation("获取服务器时间接口") |
|
||||
@PostMapping("/ServerTime") |
|
||||
public HashMap<String, Object> ServerTime(HttpServletRequest request) { |
|
||||
final String deviceId = request.getHeader("Device-ID"); // 设备ID
|
|
||||
final String now = DateUtil.format(DateUtil.date(), "yyyyMMddHHmmss") + (DateUtil.thisDayOfWeek() - 1); // 服务器时间
|
|
||||
System.out.println(deviceId + "获取服务器时间接口 = " + now); |
|
||||
HashMap<String, Object> map = new HashMap<>(); |
|
||||
map.put("Status",1); |
|
||||
map.put("Msg","服务器时间"); |
|
||||
map.put("Time", now); |
|
||||
return map; |
|
||||
} |
|
||||
|
|
||||
@ApiOperation("付款码(二维码)支付接口") |
|
||||
@PostMapping("/QRCodeTransaction") |
|
||||
public HashMap<String, Object> QRCodeTransaction(@RequestBody String QR, HttpServletRequest request) { |
|
||||
// 1.提取订单号ID
|
|
||||
final String deviceId = request.getHeader("Device-ID"); // 设备ID
|
|
||||
final JSONObject jsonObject = JSONObject.parseObject(QR); |
|
||||
final String orderNo = jsonObject.getString("QR"); |
|
||||
|
|
||||
System.out.println("deviceId = " + deviceId); |
|
||||
System.out.println("QR = " + QR); |
|
||||
|
|
||||
// 2.查询订单数据
|
|
||||
|
|
||||
// 3.查询该餐段的菜品
|
|
||||
|
|
||||
// 4.核销菜品
|
|
||||
|
|
||||
// 5.通知设备
|
|
||||
HashMap<String, Object> map = new HashMap<>(); |
|
||||
map.put("Status",1); |
|
||||
map.put("Msg","支付成功"); |
|
||||
map.put("Qrorder", orderNo); |
|
||||
|
|
||||
return map; |
|
||||
} |
|
||||
|
|
||||
@ApiOperation("二维码支付结果查询接口") |
|
||||
@PostMapping("/TransactionInquiry") |
|
||||
@Transactional(rollbackFor = {Exception.class}) |
|
||||
public HashMap<String, Object> TransactionInquiry(@RequestBody String QROrder, HttpServletRequest request) { |
|
||||
// 1. 提取订单号
|
|
||||
final JSONObject jsonObject = JSONObject.parseObject(QROrder); |
|
||||
final String orderNo = jsonObject.getString("QROrder"); |
|
||||
StringBuilder foods = new StringBuilder(); // 消费机回显信息
|
|
||||
String DeviceID = request.getHeader("Device-ID"); // 设备ID
|
|
||||
|
|
||||
final BcEquipmentParam bcEquipmentParam = new BcEquipmentParam(); |
|
||||
bcEquipmentParam.setEquipmentCode(DeviceID); |
|
||||
final List<BcEquipment> bcEquipments = bcEquipmentService.listRel(bcEquipmentParam); |
|
||||
final BcEquipment bcEquipment = bcEquipments.get(0); |
|
||||
final Integer gear = bcEquipment.getGear(); // 设备所属档口
|
|
||||
final Integer categoryId = bcUtil.getCurrentPeriod(bcEquipment.getTenantId()); // 餐段
|
|
||||
// int categoryId = 26;
|
|
||||
|
|
||||
// 打印测试
|
|
||||
System.out.println("查询支付结果(订单号:)******** = " + orderNo); |
|
||||
System.out.println("bcEquipment = " + bcEquipment); |
|
||||
System.out.println("gear = " + gear); |
|
||||
System.out.println("StrUtil.isNumeric(orderNo) = " + StrUtil.isNumeric(orderNo)); |
|
||||
|
|
||||
// 2.非代取餐模式
|
|
||||
if (!orderNo.startsWith("dqc:")) { |
|
||||
System.out.println("非代取餐模式 = "); |
|
||||
// 4.排除其他情况
|
|
||||
if(!StrUtil.isNumeric(orderNo)){ |
|
||||
foods.append("非取餐码"); |
|
||||
}else{ |
|
||||
OrderParam orderParam = new OrderParam(); |
|
||||
orderParam.setOrderNo(orderNo); |
|
||||
List<Order> list = orderService.listRel(orderParam); |
|
||||
Order order = list.get(0); |
|
||||
System.out.println("order-one = " + order); |
|
||||
OrderGoodsParam orderGoodsParam = new OrderGoodsParam(); |
|
||||
// 2.1查询订单商品
|
|
||||
List<OrderGoods> orderGoods = orderGoodsService.list(new LambdaQueryWrapper<OrderGoods>() |
|
||||
.eq(OrderGoods::getOrderId, order.getOrderId()) |
|
||||
.eq(OrderGoods::getDeliveryStatus,RECEIPT_STATUS_NO) |
|
||||
.eq(OrderGoods::getCategoryId,categoryId) |
|
||||
.eq(OrderGoods::getGear,gear) |
|
||||
); |
|
||||
// 2.2查询用户信息
|
|
||||
User user = userService.getById(order.getUserId()); |
|
||||
// 2.收集菜品信息
|
|
||||
foods.append("姓名:").append(order.getNickname()).append("\r\n"); |
|
||||
foods.append("签到:").append(DateUtil.format(DateUtil.date(),"MM-dd HH:mm")).append("\r\n"); |
|
||||
for (OrderGoods item : orderGoods) { |
|
||||
// 过滤份数totalNum=0的菜品
|
|
||||
if (!item.getTotalNum().equals(0)) { |
|
||||
foods.append(item.getGoodsName()).append(" ").append(item.getTotalNum()).append("份").append(" ¥").append(item.getTotalPrice()).append("\r\n"); |
|
||||
OrderGoods food = new OrderGoods(); |
|
||||
food.setOrderGoodsId(item.getOrderGoodsId()); |
|
||||
food.setDeliveryStatus(ORDER_GOODS_DELIVERY_STATUS20); |
|
||||
orderGoodsService.updateById(food); |
|
||||
// 更新订单部分发布状态
|
|
||||
final Order order1 = new Order(); |
|
||||
order1.setOrderId(item.getOrderId()); |
|
||||
if(categoryId == 27){ |
|
||||
order1.setDeliveryStatus(DELIVERY_STATUS_YES); |
|
||||
}else{ |
|
||||
order1.setDeliveryStatus(DELIVERY_STATUS_30); |
|
||||
} |
|
||||
orderService.updateById(order1); |
|
||||
} |
|
||||
} |
|
||||
if(orderGoods.size() == 0){ |
|
||||
foods.append("没有取餐数据"); |
|
||||
} |
|
||||
} |
|
||||
} |
|
||||
|
|
||||
// 3.代取餐模式
|
|
||||
if(orderNo.startsWith("dqc:")){ |
|
||||
System.out.println("代取餐模式 = "); |
|
||||
final String dealerId = StrUtil.removePrefix(orderNo, "dqc:"); |
|
||||
System.out.println("dealerId = " + dealerId); |
|
||||
UserRefereeParam userRefereeParam = new UserRefereeParam(); |
|
||||
userRefereeParam.setDealerId(Integer.valueOf(dealerId)); |
|
||||
userRefereeParam.setDeleted(0); |
|
||||
List<UserReferee> userRefereeList = userRefereeService.listRel(userRefereeParam); |
|
||||
System.out.println("userRefereeList = " + userRefereeList); |
|
||||
// 查询帮代餐的成员ID集
|
|
||||
List<Integer> userIds = userRefereeList.stream().map(UserReferee::getUserId).collect(Collectors.toList()); |
|
||||
System.out.println("userIds = " + userIds); |
|
||||
// 查询成员的订单
|
|
||||
List<Order> list = orderService.list(new LambdaQueryWrapper<Order>() |
|
||||
.in(Order::getUserId, userIds) |
|
||||
.eq(Order::getDeliveryTime, DateUtil.parse(DateUtil.today())) |
|
||||
.eq(Order::getPayStatus, PAY_STATUS_SUCCESS) |
|
||||
.eq(Order::getDeliveryStatus, RECEIPT_STATUS_NO) |
|
||||
.eq(Order::getDeleted, 0) |
|
||||
); |
|
||||
System.out.println("orderList = " + list); |
|
||||
if(list.size() > 0){ |
|
||||
// 收集订单ID集
|
|
||||
List<Integer> orderIds = list.stream().map(Order::getOrderId).collect(Collectors.toList()); |
|
||||
System.out.println("orderIds = " + orderIds); |
|
||||
// 查询商品
|
|
||||
if(orderIds.size() > 0){ |
|
||||
List<OrderGoods> orderGoodsList = orderGoodsService.list(new LambdaQueryWrapper<OrderGoods>() |
|
||||
.in(OrderGoods::getOrderId, orderIds) |
|
||||
.eq(OrderGoods::getDeliveryStatus,RECEIPT_STATUS_NO) |
|
||||
.eq(OrderGoods::getCategoryId,categoryId) |
|
||||
.eq(OrderGoods::getGear,gear) |
|
||||
); |
|
||||
System.out.println("orderGoodsList = " + orderGoodsList); |
|
||||
// 查询用户信息
|
|
||||
User dealer = userService.getById(dealerId); |
|
||||
System.out.println("dealer = " + dealer); |
|
||||
foods.append("代取:").append(dealer.getNickname()).append("\r\n"); |
|
||||
foods.append("签到:").append(DateUtil.format(DateUtil.date(),"MM-dd HH:mm")).append("\r\n"); |
|
||||
// 批量核销操作
|
|
||||
for (OrderGoods og : orderGoodsList) { |
|
||||
System.out.println("是否已取餐 = " + og.getGoodsName() + og.getDeliveryStatus()); |
|
||||
System.out.println("og = " + og.getOrderGoodsId()); |
|
||||
// 过滤份数totalNum=0的菜品
|
|
||||
if (!og.getTotalNum().equals(0)) { |
|
||||
foods.append(og.getGoodsName()).append(" ").append(og.getTotalNum()).append("份").append(" ¥").append(og.getTotalPrice()).append("\r\n"); |
|
||||
OrderGoods saveModel = orderGoodsService.getById(og.getOrderGoodsId()); |
|
||||
saveModel.setDeliveryStatus(ORDER_GOODS_DELIVERY_STATUS20); |
|
||||
orderGoodsService.updateById(saveModel); |
|
||||
// 更新订单部分发货状态
|
|
||||
Order order2 = new Order(); |
|
||||
order2.setOrderId(og.getOrderId()); |
|
||||
if(categoryId == 27){ |
|
||||
order2.setDeliveryStatus(DELIVERY_STATUS_YES); |
|
||||
}else{ |
|
||||
order2.setDeliveryStatus(DELIVERY_STATUS_30); |
|
||||
} |
|
||||
orderService.updateById(order2); |
|
||||
} |
|
||||
} |
|
||||
} |
|
||||
}else{ |
|
||||
foods.append("没有取餐数据"); |
|
||||
} |
|
||||
} |
|
||||
|
|
||||
// 5.通知设备
|
|
||||
HashMap<String, Object> map = new HashMap<>(); |
|
||||
map.put("Status", 1); |
|
||||
map.put("Msg",""); |
|
||||
map.put("Name", ""); |
|
||||
map.put("CardNo", ""); |
|
||||
map.put("Money", ""); |
|
||||
map.put("Subsidy", ""); |
|
||||
map.put("Times", ""); |
|
||||
map.put("Integral", ""); |
|
||||
map.put("InTime", ""); |
|
||||
map.put("OutTime", ""); |
|
||||
map.put("CumulativeTime ", ""); |
|
||||
map.put("Amount","0.00"); |
|
||||
map.put("VoiceID",null); |
|
||||
map.put("Text", foods); |
|
||||
|
|
||||
// 5.通知设备
|
|
||||
System.out.println("通知设备 = " + map); |
|
||||
return map; |
|
||||
} |
|
||||
|
|
||||
@ApiOperation("终端查询日统计接口") |
|
||||
@PostMapping("/Counts") |
|
||||
public HashMap<String, Object> Counts(HttpServletRequest request) { |
|
||||
|
|
||||
final String deviceId = request.getHeader("Device-ID"); |
|
||||
System.out.println("deviceId = " + deviceId); |
|
||||
final Integer categoryId = bcUtil.getCurrentPeriod(10048); |
|
||||
System.out.println("categoryId = " + categoryId); |
|
||||
|
|
||||
HashMap<String, Object> map = new HashMap<>(); |
|
||||
map.put("Status",1); |
|
||||
map.put("Msg","终端查询日统计接口"); |
|
||||
map.put("Text", "" + |
|
||||
"日统计\n" + |
|
||||
"日总金额:0.00\n" + |
|
||||
"日总次数:0\n" + |
|
||||
"时段统计\n" + |
|
||||
"一时段金额:0.00\n" + |
|
||||
"一时段次数:0" + |
|
||||
""); |
|
||||
|
|
||||
// 发送消息测试
|
|
||||
bcUtil.send("您的申请已通过"); |
|
||||
return map; |
|
||||
} |
|
||||
|
|
||||
|
|
||||
|
|
||||
} |
|
Loading…
Reference in new issue