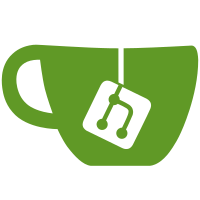
29 changed files with 1818 additions and 44 deletions
@ -0,0 +1,136 @@ |
|||
package com.gxwebsoft.tower.controller; |
|||
|
|||
import com.gxwebsoft.common.core.web.BaseController; |
|||
import com.gxwebsoft.common.system.entity.User; |
|||
import com.gxwebsoft.tower.service.TowerPartService; |
|||
import com.gxwebsoft.tower.entity.TowerPart; |
|||
import com.gxwebsoft.tower.param.TowerPartParam; |
|||
import com.gxwebsoft.common.core.web.ApiResult; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import com.gxwebsoft.common.core.web.PageParam; |
|||
import com.gxwebsoft.common.core.web.BatchParam; |
|||
import com.gxwebsoft.common.core.annotation.OperationLog; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.security.access.prepost.PreAuthorize; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import javax.annotation.Resource; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 安全检查控制器 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 18:40:31 |
|||
*/ |
|||
@Api(tags = "安全检查管理") |
|||
@RestController |
|||
@RequestMapping("/api/tower/tower-part") |
|||
public class TowerPartController extends BaseController { |
|||
@Resource |
|||
private TowerPartService towerPartService; |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPart:list')") |
|||
@OperationLog |
|||
@ApiOperation("分页查询安全检查") |
|||
@GetMapping("/page") |
|||
public ApiResult<PageResult<TowerPart>> page(TowerPartParam param) { |
|||
// 使用关联查询
|
|||
return success(towerPartService.pageRel(param)); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPart:list')") |
|||
@OperationLog |
|||
@ApiOperation("查询全部安全检查") |
|||
@GetMapping() |
|||
public ApiResult<List<TowerPart>> list(TowerPartParam param) { |
|||
PageParam<TowerPart, TowerPartParam> page = new PageParam<>(param); |
|||
page.setDefaultOrder("create_time desc"); |
|||
return success(towerPartService.list(page.getOrderWrapper())); |
|||
// 使用关联查询
|
|||
//return success(towerPartService.listRel(param));
|
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPart:list')") |
|||
@OperationLog |
|||
@ApiOperation("根据id查询安全检查") |
|||
@GetMapping("/{id}") |
|||
public ApiResult<TowerPart> get(@PathVariable("id") Integer id) { |
|||
return success(towerPartService.getById(id)); |
|||
// 使用关联查询
|
|||
//return success(towerPartService.getByIdRel(id));
|
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPart:save')") |
|||
@OperationLog |
|||
@ApiOperation("添加安全检查") |
|||
@PostMapping() |
|||
public ApiResult<?> save(@RequestBody TowerPart towerPart) { |
|||
// 记录当前登录用户id
|
|||
User loginUser = getLoginUser(); |
|||
if (loginUser != null) { |
|||
towerPart.setUserId(loginUser.getUserId()); |
|||
} |
|||
if (towerPartService.save(towerPart)) { |
|||
return success("添加成功"); |
|||
} |
|||
return fail("添加失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPart:update')") |
|||
@OperationLog |
|||
@ApiOperation("修改安全检查") |
|||
@PutMapping() |
|||
public ApiResult<?> update(@RequestBody TowerPart towerPart) { |
|||
if (towerPartService.updateById(towerPart)) { |
|||
return success("修改成功"); |
|||
} |
|||
return fail("修改失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPart:remove')") |
|||
@OperationLog |
|||
@ApiOperation("删除安全检查") |
|||
@DeleteMapping("/{id}") |
|||
public ApiResult<?> remove(@PathVariable("id") Integer id) { |
|||
if (towerPartService.removeById(id)) { |
|||
return success("删除成功"); |
|||
} |
|||
return fail("删除失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPart:save')") |
|||
@OperationLog |
|||
@ApiOperation("批量添加安全检查") |
|||
@PostMapping("/batch") |
|||
public ApiResult<?> saveBatch(@RequestBody List<TowerPart> list) { |
|||
if (towerPartService.saveBatch(list)) { |
|||
return success("添加成功"); |
|||
} |
|||
return fail("添加失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPart:update')") |
|||
@OperationLog |
|||
@ApiOperation("批量修改安全检查") |
|||
@PutMapping("/batch") |
|||
public ApiResult<?> removeBatch(@RequestBody BatchParam<TowerPart> batchParam) { |
|||
if (batchParam.update(towerPartService, "part_id")) { |
|||
return success("修改成功"); |
|||
} |
|||
return fail("修改失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPart:remove')") |
|||
@OperationLog |
|||
@ApiOperation("批量删除安全检查") |
|||
@DeleteMapping("/batch") |
|||
public ApiResult<?> removeBatch(@RequestBody List<Integer> ids) { |
|||
if (towerPartService.removeByIds(ids)) { |
|||
return success("删除成功"); |
|||
} |
|||
return fail("删除失败"); |
|||
} |
|||
|
|||
} |
@ -0,0 +1,136 @@ |
|||
package com.gxwebsoft.tower.controller; |
|||
|
|||
import com.gxwebsoft.common.core.web.BaseController; |
|||
import com.gxwebsoft.common.system.entity.User; |
|||
import com.gxwebsoft.tower.service.TowerPartGoodsService; |
|||
import com.gxwebsoft.tower.entity.TowerPartGoods; |
|||
import com.gxwebsoft.tower.param.TowerPartGoodsParam; |
|||
import com.gxwebsoft.common.core.web.ApiResult; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import com.gxwebsoft.common.core.web.PageParam; |
|||
import com.gxwebsoft.common.core.web.BatchParam; |
|||
import com.gxwebsoft.common.core.annotation.OperationLog; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.security.access.prepost.PreAuthorize; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import javax.annotation.Resource; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 安全检查控制器 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 19:41:23 |
|||
*/ |
|||
@Api(tags = "安全检查管理") |
|||
@RestController |
|||
@RequestMapping("/api/tower/tower-part-goods") |
|||
public class TowerPartGoodsController extends BaseController { |
|||
@Resource |
|||
private TowerPartGoodsService towerPartGoodsService; |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartGoods:list')") |
|||
@OperationLog |
|||
@ApiOperation("分页查询安全检查") |
|||
@GetMapping("/page") |
|||
public ApiResult<PageResult<TowerPartGoods>> page(TowerPartGoodsParam param) { |
|||
// 使用关联查询
|
|||
return success(towerPartGoodsService.pageRel(param)); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartGoods:list')") |
|||
@OperationLog |
|||
@ApiOperation("查询全部安全检查") |
|||
@GetMapping() |
|||
public ApiResult<List<TowerPartGoods>> list(TowerPartGoodsParam param) { |
|||
PageParam<TowerPartGoods, TowerPartGoodsParam> page = new PageParam<>(param); |
|||
page.setDefaultOrder("create_time desc"); |
|||
return success(towerPartGoodsService.list(page.getOrderWrapper())); |
|||
// 使用关联查询
|
|||
//return success(towerPartGoodsService.listRel(param));
|
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartGoods:list')") |
|||
@OperationLog |
|||
@ApiOperation("根据id查询安全检查") |
|||
@GetMapping("/{id}") |
|||
public ApiResult<TowerPartGoods> get(@PathVariable("id") Integer id) { |
|||
return success(towerPartGoodsService.getById(id)); |
|||
// 使用关联查询
|
|||
//return success(towerPartGoodsService.getByIdRel(id));
|
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartGoods:save')") |
|||
@OperationLog |
|||
@ApiOperation("添加安全检查") |
|||
@PostMapping() |
|||
public ApiResult<?> save(@RequestBody TowerPartGoods towerPartGoods) { |
|||
// 记录当前登录用户id
|
|||
User loginUser = getLoginUser(); |
|||
if (loginUser != null) { |
|||
towerPartGoods.setUserId(loginUser.getUserId()); |
|||
} |
|||
if (towerPartGoodsService.save(towerPartGoods)) { |
|||
return success("添加成功"); |
|||
} |
|||
return fail("添加失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartGoods:update')") |
|||
@OperationLog |
|||
@ApiOperation("修改安全检查") |
|||
@PutMapping() |
|||
public ApiResult<?> update(@RequestBody TowerPartGoods towerPartGoods) { |
|||
if (towerPartGoodsService.updateById(towerPartGoods)) { |
|||
return success("修改成功"); |
|||
} |
|||
return fail("修改失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartGoods:remove')") |
|||
@OperationLog |
|||
@ApiOperation("删除安全检查") |
|||
@DeleteMapping("/{id}") |
|||
public ApiResult<?> remove(@PathVariable("id") Integer id) { |
|||
if (towerPartGoodsService.removeById(id)) { |
|||
return success("删除成功"); |
|||
} |
|||
return fail("删除失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartGoods:save')") |
|||
@OperationLog |
|||
@ApiOperation("批量添加安全检查") |
|||
@PostMapping("/batch") |
|||
public ApiResult<?> saveBatch(@RequestBody List<TowerPartGoods> list) { |
|||
if (towerPartGoodsService.saveBatch(list)) { |
|||
return success("添加成功"); |
|||
} |
|||
return fail("添加失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartGoods:update')") |
|||
@OperationLog |
|||
@ApiOperation("批量修改安全检查") |
|||
@PutMapping("/batch") |
|||
public ApiResult<?> removeBatch(@RequestBody BatchParam<TowerPartGoods> batchParam) { |
|||
if (batchParam.update(towerPartGoodsService, "goods_id")) { |
|||
return success("修改成功"); |
|||
} |
|||
return fail("修改失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartGoods:remove')") |
|||
@OperationLog |
|||
@ApiOperation("批量删除安全检查") |
|||
@DeleteMapping("/batch") |
|||
public ApiResult<?> removeBatch(@RequestBody List<Integer> ids) { |
|||
if (towerPartGoodsService.removeByIds(ids)) { |
|||
return success("删除成功"); |
|||
} |
|||
return fail("删除失败"); |
|||
} |
|||
|
|||
} |
@ -0,0 +1,136 @@ |
|||
package com.gxwebsoft.tower.controller; |
|||
|
|||
import com.gxwebsoft.common.core.web.BaseController; |
|||
import com.gxwebsoft.common.system.entity.User; |
|||
import com.gxwebsoft.tower.service.TowerPartStockService; |
|||
import com.gxwebsoft.tower.entity.TowerPartStock; |
|||
import com.gxwebsoft.tower.param.TowerPartStockParam; |
|||
import com.gxwebsoft.common.core.web.ApiResult; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import com.gxwebsoft.common.core.web.PageParam; |
|||
import com.gxwebsoft.common.core.web.BatchParam; |
|||
import com.gxwebsoft.common.core.annotation.OperationLog; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.security.access.prepost.PreAuthorize; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import javax.annotation.Resource; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 产品库存控制器 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 19:41:23 |
|||
*/ |
|||
@Api(tags = "产品库存管理") |
|||
@RestController |
|||
@RequestMapping("/api/tower/tower-part-stock") |
|||
public class TowerPartStockController extends BaseController { |
|||
@Resource |
|||
private TowerPartStockService towerPartStockService; |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartStock:list')") |
|||
@OperationLog |
|||
@ApiOperation("分页查询产品库存") |
|||
@GetMapping("/page") |
|||
public ApiResult<PageResult<TowerPartStock>> page(TowerPartStockParam param) { |
|||
// 使用关联查询
|
|||
return success(towerPartStockService.pageRel(param)); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartStock:list')") |
|||
@OperationLog |
|||
@ApiOperation("查询全部产品库存") |
|||
@GetMapping() |
|||
public ApiResult<List<TowerPartStock>> list(TowerPartStockParam param) { |
|||
PageParam<TowerPartStock, TowerPartStockParam> page = new PageParam<>(param); |
|||
page.setDefaultOrder("create_time desc"); |
|||
return success(towerPartStockService.list(page.getOrderWrapper())); |
|||
// 使用关联查询
|
|||
//return success(towerPartStockService.listRel(param));
|
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartStock:list')") |
|||
@OperationLog |
|||
@ApiOperation("根据id查询产品库存") |
|||
@GetMapping("/{id}") |
|||
public ApiResult<TowerPartStock> get(@PathVariable("id") Integer id) { |
|||
return success(towerPartStockService.getById(id)); |
|||
// 使用关联查询
|
|||
//return success(towerPartStockService.getByIdRel(id));
|
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartStock:save')") |
|||
@OperationLog |
|||
@ApiOperation("添加产品库存") |
|||
@PostMapping() |
|||
public ApiResult<?> save(@RequestBody TowerPartStock towerPartStock) { |
|||
// 记录当前登录用户id
|
|||
User loginUser = getLoginUser(); |
|||
if (loginUser != null) { |
|||
towerPartStock.setUserId(loginUser.getUserId()); |
|||
} |
|||
if (towerPartStockService.save(towerPartStock)) { |
|||
return success("添加成功"); |
|||
} |
|||
return fail("添加失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartStock:update')") |
|||
@OperationLog |
|||
@ApiOperation("修改产品库存") |
|||
@PutMapping() |
|||
public ApiResult<?> update(@RequestBody TowerPartStock towerPartStock) { |
|||
if (towerPartStockService.updateById(towerPartStock)) { |
|||
return success("修改成功"); |
|||
} |
|||
return fail("修改失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartStock:remove')") |
|||
@OperationLog |
|||
@ApiOperation("删除产品库存") |
|||
@DeleteMapping("/{id}") |
|||
public ApiResult<?> remove(@PathVariable("id") Integer id) { |
|||
if (towerPartStockService.removeById(id)) { |
|||
return success("删除成功"); |
|||
} |
|||
return fail("删除失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartStock:save')") |
|||
@OperationLog |
|||
@ApiOperation("批量添加产品库存") |
|||
@PostMapping("/batch") |
|||
public ApiResult<?> saveBatch(@RequestBody List<TowerPartStock> list) { |
|||
if (towerPartStockService.saveBatch(list)) { |
|||
return success("添加成功"); |
|||
} |
|||
return fail("添加失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartStock:update')") |
|||
@OperationLog |
|||
@ApiOperation("批量修改产品库存") |
|||
@PutMapping("/batch") |
|||
public ApiResult<?> removeBatch(@RequestBody BatchParam<TowerPartStock> batchParam) { |
|||
if (batchParam.update(towerPartStockService, "part_id")) { |
|||
return success("修改成功"); |
|||
} |
|||
return fail("修改失败"); |
|||
} |
|||
|
|||
@PreAuthorize("hasAuthority('tower:towerPartStock:remove')") |
|||
@OperationLog |
|||
@ApiOperation("批量删除产品库存") |
|||
@DeleteMapping("/batch") |
|||
public ApiResult<?> removeBatch(@RequestBody List<Integer> ids) { |
|||
if (towerPartStockService.removeByIds(ids)) { |
|||
return success("删除成功"); |
|||
} |
|||
return fail("删除失败"); |
|||
} |
|||
|
|||
} |
@ -0,0 +1,84 @@ |
|||
package com.gxwebsoft.tower.entity; |
|||
|
|||
import java.math.BigDecimal; |
|||
import com.baomidou.mybatisplus.annotation.IdType; |
|||
import com.baomidou.mybatisplus.annotation.TableId; |
|||
import java.time.LocalDateTime; |
|||
import com.baomidou.mybatisplus.annotation.TableLogic; |
|||
import java.io.Serializable; |
|||
import java.util.Date; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
import lombok.EqualsAndHashCode; |
|||
|
|||
/** |
|||
* 安全检查 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 18:40:31 |
|||
*/ |
|||
@Data |
|||
@EqualsAndHashCode(callSuper = false) |
|||
@ApiModel(value = "TowerPart对象", description = "安全检查") |
|||
public class TowerPart implements Serializable { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty(value = "自增ID") |
|||
@TableId(value = "part_id", type = IdType.AUTO) |
|||
private Integer partId; |
|||
|
|||
@ApiModelProperty(value = "配件编码") |
|||
private String partCode; |
|||
|
|||
@ApiModelProperty(value = "类别") |
|||
private String category; |
|||
|
|||
@ApiModelProperty(value = "规格") |
|||
private String specs; |
|||
|
|||
@ApiModelProperty(value = "位置") |
|||
private String position; |
|||
|
|||
@ApiModelProperty(value = "库存数量") |
|||
private Integer stock; |
|||
|
|||
@ApiModelProperty(value = "库存金额") |
|||
private BigDecimal stockMoney; |
|||
|
|||
@ApiModelProperty(value = "进货价格") |
|||
private BigDecimal price; |
|||
|
|||
@ApiModelProperty(value = "最低库存") |
|||
private Integer stockMin; |
|||
|
|||
@ApiModelProperty(value = "是否低于最低库存") |
|||
private Integer stockLower; |
|||
|
|||
@ApiModelProperty(value = "状态, 0正常, 1待修,2异常已修,3异常未修") |
|||
private Integer status; |
|||
|
|||
@ApiModelProperty(value = "备注") |
|||
private String comments; |
|||
|
|||
@ApiModelProperty(value = "排序号") |
|||
private Integer sortNumber; |
|||
|
|||
@ApiModelProperty(value = "所有人") |
|||
private Integer userId; |
|||
|
|||
@ApiModelProperty(value = "是否删除, 0否, 1是") |
|||
@TableLogic |
|||
private Integer deleted; |
|||
|
|||
@ApiModelProperty(value = "租户id") |
|||
private Integer tenantId; |
|||
|
|||
@ApiModelProperty(value = "创建时间") |
|||
private Date createTime; |
|||
|
|||
@ApiModelProperty(value = "修改时间") |
|||
private Date updateTime; |
|||
|
|||
} |
@ -0,0 +1,107 @@ |
|||
package com.gxwebsoft.tower.entity; |
|||
|
|||
import java.math.BigDecimal; |
|||
import com.baomidou.mybatisplus.annotation.IdType; |
|||
import com.baomidou.mybatisplus.annotation.TableField; |
|||
import com.baomidou.mybatisplus.annotation.TableId; |
|||
import java.time.LocalDateTime; |
|||
import com.baomidou.mybatisplus.annotation.TableLogic; |
|||
import java.io.Serializable; |
|||
import java.util.Date; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
import lombok.EqualsAndHashCode; |
|||
|
|||
/** |
|||
* 安全检查 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 19:41:23 |
|||
*/ |
|||
@Data |
|||
@EqualsAndHashCode(callSuper = false) |
|||
@ApiModel(value = "TowerPartGoods对象", description = "安全检查") |
|||
public class TowerPartGoods implements Serializable { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty(value = "自增ID") |
|||
@TableId(value = "goods_id", type = IdType.AUTO) |
|||
private Integer goodsId; |
|||
|
|||
@ApiModelProperty(value = "配件编码") |
|||
private String goodsCode; |
|||
|
|||
@ApiModelProperty(value = "类别") |
|||
private String category; |
|||
|
|||
@ApiModelProperty(value = "产品名称") |
|||
private String goodsName; |
|||
|
|||
@ApiModelProperty(value = "规格") |
|||
private String specs; |
|||
|
|||
@ApiModelProperty(value = "货架") |
|||
private String position; |
|||
|
|||
@ApiModelProperty(value = "进货价格") |
|||
private BigDecimal price; |
|||
|
|||
@ApiModelProperty(value = "销售价格") |
|||
private BigDecimal salePrice; |
|||
|
|||
@ApiModelProperty(value = "状态, 0正常, 1待修,2异常已修,3异常未修") |
|||
private Integer status; |
|||
|
|||
@ApiModelProperty(value = "备注") |
|||
private String comments; |
|||
|
|||
@ApiModelProperty(value = "排序号") |
|||
private Integer sortNumber; |
|||
|
|||
@ApiModelProperty(value = "所有人") |
|||
private Integer userId; |
|||
|
|||
@ApiModelProperty(value = "是否删除, 0否, 1是") |
|||
@TableLogic |
|||
private Integer deleted; |
|||
|
|||
@ApiModelProperty(value = "租户id") |
|||
private Integer tenantId; |
|||
|
|||
@ApiModelProperty(value = "创建时间") |
|||
private Date createTime; |
|||
|
|||
@ApiModelProperty(value = "修改时间") |
|||
private Date updateTime; |
|||
|
|||
@ApiModelProperty(value = "昵称") |
|||
@TableField(exist = false) |
|||
private String nickname; |
|||
|
|||
@ApiModelProperty(value = "手机号码") |
|||
@TableField(exist = false) |
|||
private String phone; |
|||
|
|||
@ApiModelProperty(value = "头像") |
|||
@TableField(exist = false) |
|||
private String avatar; |
|||
|
|||
@ApiModelProperty(value = "库存数量") |
|||
@TableField(exist = false) |
|||
private String stock; |
|||
|
|||
@ApiModelProperty(value = "库存金额") |
|||
@TableField(exist = false) |
|||
private BigDecimal stockMoney; |
|||
|
|||
@ApiModelProperty(value = "最低库存") |
|||
@TableField(exist = false) |
|||
private Integer stockMin; |
|||
|
|||
@ApiModelProperty(value = "是否低于最低库存") |
|||
@TableField(exist = false) |
|||
private Integer stockLower; |
|||
|
|||
} |
@ -0,0 +1,99 @@ |
|||
package com.gxwebsoft.tower.entity; |
|||
|
|||
import java.math.BigDecimal; |
|||
import com.baomidou.mybatisplus.annotation.IdType; |
|||
import com.baomidou.mybatisplus.annotation.TableField; |
|||
import com.baomidou.mybatisplus.annotation.TableId; |
|||
import com.baomidou.mybatisplus.annotation.TableLogic; |
|||
import java.io.Serializable; |
|||
import java.util.Date; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
import lombok.EqualsAndHashCode; |
|||
|
|||
/** |
|||
* 产品库存 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 19:41:23 |
|||
*/ |
|||
@Data |
|||
@EqualsAndHashCode(callSuper = false) |
|||
@ApiModel(value = "TowerPartStock对象", description = "产品库存") |
|||
public class TowerPartStock implements Serializable { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty(value = "自增ID") |
|||
@TableId(value = "part_id", type = IdType.AUTO) |
|||
private Integer partId; |
|||
|
|||
@ApiModelProperty(value = "产品ID") |
|||
private Integer goodsId; |
|||
|
|||
@ApiModelProperty(value = "配件编码") |
|||
private String partCode; |
|||
|
|||
@ApiModelProperty(value = "类别") |
|||
private String category; |
|||
|
|||
@ApiModelProperty(value = "规格") |
|||
private String specs; |
|||
|
|||
@ApiModelProperty(value = "位置") |
|||
private String position; |
|||
|
|||
@ApiModelProperty(value = "库存数量") |
|||
private Integer stock; |
|||
|
|||
@ApiModelProperty(value = "库存金额") |
|||
private BigDecimal stockMoney; |
|||
|
|||
@ApiModelProperty(value = "进货价格") |
|||
private BigDecimal price; |
|||
|
|||
@ApiModelProperty(value = "最低库存") |
|||
private Integer stockMin; |
|||
|
|||
@ApiModelProperty(value = "是否低于最低库存") |
|||
private Integer stockLower; |
|||
|
|||
@ApiModelProperty(value = "状态, 0正常, 1待修,2异常已修,3异常未修") |
|||
private Integer status; |
|||
|
|||
@ApiModelProperty(value = "备注") |
|||
private String comments; |
|||
|
|||
@ApiModelProperty(value = "排序号") |
|||
private Integer sortNumber; |
|||
|
|||
@ApiModelProperty(value = "所有人") |
|||
private Integer userId; |
|||
|
|||
@ApiModelProperty(value = "是否删除, 0否, 1是") |
|||
@TableLogic |
|||
private Integer deleted; |
|||
|
|||
@ApiModelProperty(value = "租户id") |
|||
private Integer tenantId; |
|||
|
|||
@ApiModelProperty(value = "创建时间") |
|||
private Date createTime; |
|||
|
|||
@ApiModelProperty(value = "修改时间") |
|||
private Date updateTime; |
|||
|
|||
@ApiModelProperty(value = "昵称") |
|||
@TableField(exist = false) |
|||
private String nickname; |
|||
|
|||
@ApiModelProperty(value = "手机号码") |
|||
@TableField(exist = false) |
|||
private String phone; |
|||
|
|||
@ApiModelProperty(value = "头像") |
|||
@TableField(exist = false) |
|||
private String avatar; |
|||
|
|||
} |
@ -0,0 +1,37 @@ |
|||
package com.gxwebsoft.tower.mapper; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.gxwebsoft.tower.entity.TowerPartGoods; |
|||
import com.gxwebsoft.tower.param.TowerPartGoodsParam; |
|||
import org.apache.ibatis.annotations.Param; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 安全检查Mapper |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 19:41:23 |
|||
*/ |
|||
public interface TowerPartGoodsMapper extends BaseMapper<TowerPartGoods> { |
|||
|
|||
/** |
|||
* 分页查询 |
|||
* |
|||
* @param page 分页对象 |
|||
* @param param 查询参数 |
|||
* @return List<TowerPartGoods> |
|||
*/ |
|||
List<TowerPartGoods> selectPageRel(@Param("page") IPage<TowerPartGoods> page, |
|||
@Param("param") TowerPartGoodsParam param); |
|||
|
|||
/** |
|||
* 查询全部 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return List<User> |
|||
*/ |
|||
List<TowerPartGoods> selectListRel(@Param("param") TowerPartGoodsParam param); |
|||
|
|||
} |
@ -0,0 +1,37 @@ |
|||
package com.gxwebsoft.tower.mapper; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.gxwebsoft.tower.entity.TowerPart; |
|||
import com.gxwebsoft.tower.param.TowerPartParam; |
|||
import org.apache.ibatis.annotations.Param; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 安全检查Mapper |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 18:40:31 |
|||
*/ |
|||
public interface TowerPartMapper extends BaseMapper<TowerPart> { |
|||
|
|||
/** |
|||
* 分页查询 |
|||
* |
|||
* @param page 分页对象 |
|||
* @param param 查询参数 |
|||
* @return List<TowerPart> |
|||
*/ |
|||
List<TowerPart> selectPageRel(@Param("page") IPage<TowerPart> page, |
|||
@Param("param") TowerPartParam param); |
|||
|
|||
/** |
|||
* 查询全部 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return List<User> |
|||
*/ |
|||
List<TowerPart> selectListRel(@Param("param") TowerPartParam param); |
|||
|
|||
} |
@ -0,0 +1,37 @@ |
|||
package com.gxwebsoft.tower.mapper; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.gxwebsoft.tower.entity.TowerPartStock; |
|||
import com.gxwebsoft.tower.param.TowerPartStockParam; |
|||
import org.apache.ibatis.annotations.Param; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 产品库存Mapper |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 19:41:23 |
|||
*/ |
|||
public interface TowerPartStockMapper extends BaseMapper<TowerPartStock> { |
|||
|
|||
/** |
|||
* 分页查询 |
|||
* |
|||
* @param page 分页对象 |
|||
* @param param 查询参数 |
|||
* @return List<TowerPartStock> |
|||
*/ |
|||
List<TowerPartStock> selectPageRel(@Param("page") IPage<TowerPartStock> page, |
|||
@Param("param") TowerPartStockParam param); |
|||
|
|||
/** |
|||
* 查询全部 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return List<User> |
|||
*/ |
|||
List<TowerPartStock> selectListRel(@Param("param") TowerPartStockParam param); |
|||
|
|||
} |
@ -0,0 +1,76 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.gxwebsoft.tower.mapper.TowerPartGoodsMapper"> |
|||
|
|||
<!-- 关联查询sql --> |
|||
<sql id="selectSql"> |
|||
SELECT a.*,b.nickname,b.phone,b.avatar,c.stock,c.stock_money,c.stock_min,c.stock_lower |
|||
FROM tower_part_goods a |
|||
LEFT JOIN sys_user b ON a.user_id = b.user_id |
|||
LEFT JOIN tower_part_stock c ON a.goods_id = c.goods_id |
|||
<where> |
|||
<if test="param.goodsId != null"> |
|||
AND a.goods_id = #{param.goodsId} |
|||
</if> |
|||
<if test="param.goodsCode != null"> |
|||
AND a.goods_code LIKE CONCAT('%', #{param.goodsCode}, '%') |
|||
</if> |
|||
<if test="param.category != null"> |
|||
AND a.category LIKE CONCAT('%', #{param.category}, '%') |
|||
</if> |
|||
<if test="param.specs != null"> |
|||
AND a.specs LIKE CONCAT('%', #{param.specs}, '%') |
|||
</if> |
|||
<if test="param.position != null"> |
|||
AND a.position LIKE CONCAT('%', #{param.position}, '%') |
|||
</if> |
|||
<if test="param.price != null"> |
|||
AND a.price = #{param.price} |
|||
</if> |
|||
<if test="param.salePrice != null"> |
|||
AND a.sale_price = #{param.salePrice} |
|||
</if> |
|||
<if test="param.status != null"> |
|||
AND a.status = #{param.status} |
|||
</if> |
|||
<if test="param.comments != null"> |
|||
AND a.comments LIKE CONCAT('%', #{param.comments}, '%') |
|||
</if> |
|||
<if test="param.sortNumber != null"> |
|||
AND a.sort_number = #{param.sortNumber} |
|||
</if> |
|||
<if test="param.userId != null"> |
|||
AND a.user_id = #{param.userId} |
|||
</if> |
|||
<if test="param.deleted != null"> |
|||
AND a.deleted = #{param.deleted} |
|||
</if> |
|||
<if test="param.deleted == null"> |
|||
AND a.deleted = 0 |
|||
</if> |
|||
<if test="param.createTimeStart != null"> |
|||
AND a.create_time >= #{param.createTimeStart} |
|||
</if> |
|||
<if test="param.createTimeEnd != null"> |
|||
AND a.create_time <= #{param.createTimeEnd} |
|||
</if> |
|||
<if test="param.keywords != null"> |
|||
AND (a.goods_name LIKE CONCAT('%', #{param.keywords}, '%') |
|||
OR a.goods_id = #{param.keywords} |
|||
OR a.goods_code = #{param.keywords} |
|||
) |
|||
</if> |
|||
</where> |
|||
</sql> |
|||
|
|||
<!-- 分页查询 --> |
|||
<select id="selectPageRel" resultType="com.gxwebsoft.tower.entity.TowerPartGoods"> |
|||
<include refid="selectSql"></include> |
|||
</select> |
|||
|
|||
<!-- 查询全部 --> |
|||
<select id="selectListRel" resultType="com.gxwebsoft.tower.entity.TowerPartGoods"> |
|||
<include refid="selectSql"></include> |
|||
</select> |
|||
|
|||
</mapper> |
@ -0,0 +1,77 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.gxwebsoft.tower.mapper.TowerPartMapper"> |
|||
|
|||
<!-- 关联查询sql --> |
|||
<sql id="selectSql"> |
|||
SELECT a.* |
|||
FROM tower_part a |
|||
<where> |
|||
<if test="param.partId != null"> |
|||
AND a.part_id = #{param.partId} |
|||
</if> |
|||
<if test="param.partCode != null"> |
|||
AND a.part_code LIKE CONCAT('%', #{param.partCode}, '%') |
|||
</if> |
|||
<if test="param.category != null"> |
|||
AND a.category LIKE CONCAT('%', #{param.category}, '%') |
|||
</if> |
|||
<if test="param.specs != null"> |
|||
AND a.specs LIKE CONCAT('%', #{param.specs}, '%') |
|||
</if> |
|||
<if test="param.position != null"> |
|||
AND a.position LIKE CONCAT('%', #{param.position}, '%') |
|||
</if> |
|||
<if test="param.stock != null"> |
|||
AND a.stock = #{param.stock} |
|||
</if> |
|||
<if test="param.stockMoney != null"> |
|||
AND a.stock_money = #{param.stockMoney} |
|||
</if> |
|||
<if test="param.price != null"> |
|||
AND a.price = #{param.price} |
|||
</if> |
|||
<if test="param.stockMin != null"> |
|||
AND a.stock_min = #{param.stockMin} |
|||
</if> |
|||
<if test="param.stockLower != null"> |
|||
AND a.stock_lower = #{param.stockLower} |
|||
</if> |
|||
<if test="param.status != null"> |
|||
AND a.status = #{param.status} |
|||
</if> |
|||
<if test="param.comments != null"> |
|||
AND a.comments LIKE CONCAT('%', #{param.comments}, '%') |
|||
</if> |
|||
<if test="param.sortNumber != null"> |
|||
AND a.sort_number = #{param.sortNumber} |
|||
</if> |
|||
<if test="param.userId != null"> |
|||
AND a.user_id = #{param.userId} |
|||
</if> |
|||
<if test="param.deleted != null"> |
|||
AND a.deleted = #{param.deleted} |
|||
</if> |
|||
<if test="param.deleted == null"> |
|||
AND a.deleted = 0 |
|||
</if> |
|||
<if test="param.createTimeStart != null"> |
|||
AND a.create_time >= #{param.createTimeStart} |
|||
</if> |
|||
<if test="param.createTimeEnd != null"> |
|||
AND a.create_time <= #{param.createTimeEnd} |
|||
</if> |
|||
</where> |
|||
</sql> |
|||
|
|||
<!-- 分页查询 --> |
|||
<select id="selectPageRel" resultType="com.gxwebsoft.tower.entity.TowerPart"> |
|||
<include refid="selectSql"></include> |
|||
</select> |
|||
|
|||
<!-- 查询全部 --> |
|||
<select id="selectListRel" resultType="com.gxwebsoft.tower.entity.TowerPart"> |
|||
<include refid="selectSql"></include> |
|||
</select> |
|||
|
|||
</mapper> |
@ -0,0 +1,81 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.gxwebsoft.tower.mapper.TowerPartStockMapper"> |
|||
|
|||
<!-- 关联查询sql --> |
|||
<sql id="selectSql"> |
|||
SELECT a.*,b.nickname,b.phone,b.avatar |
|||
FROM tower_part_stock a |
|||
LEFT JOIN sys_user b ON a.user_id = b.user_id |
|||
<where> |
|||
<if test="param.partId != null"> |
|||
AND a.part_id = #{param.partId} |
|||
</if> |
|||
<if test="param.goodsId != null"> |
|||
AND a.goods_id = #{param.goodsId} |
|||
</if> |
|||
<if test="param.partCode != null"> |
|||
AND a.part_code LIKE CONCAT('%', #{param.partCode}, '%') |
|||
</if> |
|||
<if test="param.category != null"> |
|||
AND a.category LIKE CONCAT('%', #{param.category}, '%') |
|||
</if> |
|||
<if test="param.specs != null"> |
|||
AND a.specs LIKE CONCAT('%', #{param.specs}, '%') |
|||
</if> |
|||
<if test="param.position != null"> |
|||
AND a.position LIKE CONCAT('%', #{param.position}, '%') |
|||
</if> |
|||
<if test="param.stock != null"> |
|||
AND a.stock = #{param.stock} |
|||
</if> |
|||
<if test="param.stockMoney != null"> |
|||
AND a.stock_money = #{param.stockMoney} |
|||
</if> |
|||
<if test="param.price != null"> |
|||
AND a.price = #{param.price} |
|||
</if> |
|||
<if test="param.stockMin != null"> |
|||
AND a.stock_min = #{param.stockMin} |
|||
</if> |
|||
<if test="param.stockLower != null"> |
|||
AND a.stock_lower = #{param.stockLower} |
|||
</if> |
|||
<if test="param.status != null"> |
|||
AND a.status = #{param.status} |
|||
</if> |
|||
<if test="param.comments != null"> |
|||
AND a.comments LIKE CONCAT('%', #{param.comments}, '%') |
|||
</if> |
|||
<if test="param.sortNumber != null"> |
|||
AND a.sort_number = #{param.sortNumber} |
|||
</if> |
|||
<if test="param.userId != null"> |
|||
AND a.user_id = #{param.userId} |
|||
</if> |
|||
<if test="param.deleted != null"> |
|||
AND a.deleted = #{param.deleted} |
|||
</if> |
|||
<if test="param.deleted == null"> |
|||
AND a.deleted = 0 |
|||
</if> |
|||
<if test="param.createTimeStart != null"> |
|||
AND a.create_time >= #{param.createTimeStart} |
|||
</if> |
|||
<if test="param.createTimeEnd != null"> |
|||
AND a.create_time <= #{param.createTimeEnd} |
|||
</if> |
|||
</where> |
|||
</sql> |
|||
|
|||
<!-- 分页查询 --> |
|||
<select id="selectPageRel" resultType="com.gxwebsoft.tower.entity.TowerPartStock"> |
|||
<include refid="selectSql"></include> |
|||
</select> |
|||
|
|||
<!-- 查询全部 --> |
|||
<select id="selectListRel" resultType="com.gxwebsoft.tower.entity.TowerPartStock"> |
|||
<include refid="selectSql"></include> |
|||
</select> |
|||
|
|||
</mapper> |
@ -0,0 +1,70 @@ |
|||
package com.gxwebsoft.tower.param; |
|||
|
|||
import com.gxwebsoft.common.core.annotation.QueryField; |
|||
import com.gxwebsoft.common.core.annotation.QueryType; |
|||
import com.gxwebsoft.common.core.web.BaseParam; |
|||
import com.fasterxml.jackson.annotation.JsonInclude; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
import lombok.EqualsAndHashCode; |
|||
|
|||
import java.math.BigDecimal; |
|||
|
|||
/** |
|||
* 安全检查查询参数 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 19:41:23 |
|||
*/ |
|||
@Data |
|||
@EqualsAndHashCode(callSuper = false) |
|||
@JsonInclude(JsonInclude.Include.NON_NULL) |
|||
@ApiModel(value = "TowerPartGoodsParam对象", description = "安全检查查询参数") |
|||
public class TowerPartGoodsParam extends BaseParam { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty(value = "自增ID") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer goodsId; |
|||
|
|||
@ApiModelProperty(value = "配件编码") |
|||
private String goodsCode; |
|||
|
|||
@ApiModelProperty(value = "类别") |
|||
private String category; |
|||
|
|||
@ApiModelProperty(value = "规格") |
|||
private String specs; |
|||
|
|||
@ApiModelProperty(value = "货架") |
|||
private String position; |
|||
|
|||
@ApiModelProperty(value = "进货价格") |
|||
@QueryField(type = QueryType.EQ) |
|||
private BigDecimal price; |
|||
|
|||
@ApiModelProperty(value = "销售价格") |
|||
@QueryField(type = QueryType.EQ) |
|||
private BigDecimal salePrice; |
|||
|
|||
@ApiModelProperty(value = "状态, 0正常, 1待修,2异常已修,3异常未修") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer status; |
|||
|
|||
@ApiModelProperty(value = "备注") |
|||
private String comments; |
|||
|
|||
@ApiModelProperty(value = "排序号") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer sortNumber; |
|||
|
|||
@ApiModelProperty(value = "所有人") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer userId; |
|||
|
|||
@ApiModelProperty(value = "是否删除, 0否, 1是") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer deleted; |
|||
|
|||
} |
@ -0,0 +1,82 @@ |
|||
package com.gxwebsoft.tower.param; |
|||
|
|||
import com.gxwebsoft.common.core.annotation.QueryField; |
|||
import com.gxwebsoft.common.core.annotation.QueryType; |
|||
import com.gxwebsoft.common.core.web.BaseParam; |
|||
import com.fasterxml.jackson.annotation.JsonInclude; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
import lombok.EqualsAndHashCode; |
|||
|
|||
import java.math.BigDecimal; |
|||
|
|||
/** |
|||
* 安全检查查询参数 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 18:40:31 |
|||
*/ |
|||
@Data |
|||
@EqualsAndHashCode(callSuper = false) |
|||
@JsonInclude(JsonInclude.Include.NON_NULL) |
|||
@ApiModel(value = "TowerPartParam对象", description = "安全检查查询参数") |
|||
public class TowerPartParam extends BaseParam { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty(value = "自增ID") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer partId; |
|||
|
|||
@ApiModelProperty(value = "配件编码") |
|||
private String partCode; |
|||
|
|||
@ApiModelProperty(value = "类别") |
|||
private String category; |
|||
|
|||
@ApiModelProperty(value = "规格") |
|||
private String specs; |
|||
|
|||
@ApiModelProperty(value = "位置") |
|||
private String position; |
|||
|
|||
@ApiModelProperty(value = "库存数量") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer stock; |
|||
|
|||
@ApiModelProperty(value = "库存金额") |
|||
@QueryField(type = QueryType.EQ) |
|||
private BigDecimal stockMoney; |
|||
|
|||
@ApiModelProperty(value = "进货价格") |
|||
@QueryField(type = QueryType.EQ) |
|||
private BigDecimal price; |
|||
|
|||
@ApiModelProperty(value = "最低库存") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer stockMin; |
|||
|
|||
@ApiModelProperty(value = "是否低于最低库存") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer stockLower; |
|||
|
|||
@ApiModelProperty(value = "状态, 0正常, 1待修,2异常已修,3异常未修") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer status; |
|||
|
|||
@ApiModelProperty(value = "备注") |
|||
private String comments; |
|||
|
|||
@ApiModelProperty(value = "排序号") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer sortNumber; |
|||
|
|||
@ApiModelProperty(value = "所有人") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer userId; |
|||
|
|||
@ApiModelProperty(value = "是否删除, 0否, 1是") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer deleted; |
|||
|
|||
} |
@ -0,0 +1,86 @@ |
|||
package com.gxwebsoft.tower.param; |
|||
|
|||
import com.gxwebsoft.common.core.annotation.QueryField; |
|||
import com.gxwebsoft.common.core.annotation.QueryType; |
|||
import com.gxwebsoft.common.core.web.BaseParam; |
|||
import com.fasterxml.jackson.annotation.JsonInclude; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
import lombok.EqualsAndHashCode; |
|||
|
|||
import java.math.BigDecimal; |
|||
|
|||
/** |
|||
* 产品库存查询参数 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 19:41:23 |
|||
*/ |
|||
@Data |
|||
@EqualsAndHashCode(callSuper = false) |
|||
@JsonInclude(JsonInclude.Include.NON_NULL) |
|||
@ApiModel(value = "TowerPartStockParam对象", description = "产品库存查询参数") |
|||
public class TowerPartStockParam extends BaseParam { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty(value = "自增ID") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer partId; |
|||
|
|||
@ApiModelProperty(value = "产品ID") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer goodsId; |
|||
|
|||
@ApiModelProperty(value = "配件编码") |
|||
private String partCode; |
|||
|
|||
@ApiModelProperty(value = "类别") |
|||
private String category; |
|||
|
|||
@ApiModelProperty(value = "规格") |
|||
private String specs; |
|||
|
|||
@ApiModelProperty(value = "位置") |
|||
private String position; |
|||
|
|||
@ApiModelProperty(value = "库存数量") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer stock; |
|||
|
|||
@ApiModelProperty(value = "库存金额") |
|||
@QueryField(type = QueryType.EQ) |
|||
private BigDecimal stockMoney; |
|||
|
|||
@ApiModelProperty(value = "进货价格") |
|||
@QueryField(type = QueryType.EQ) |
|||
private BigDecimal price; |
|||
|
|||
@ApiModelProperty(value = "最低库存") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer stockMin; |
|||
|
|||
@ApiModelProperty(value = "是否低于最低库存") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer stockLower; |
|||
|
|||
@ApiModelProperty(value = "状态, 0正常, 1待修,2异常已修,3异常未修") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer status; |
|||
|
|||
@ApiModelProperty(value = "备注") |
|||
private String comments; |
|||
|
|||
@ApiModelProperty(value = "排序号") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer sortNumber; |
|||
|
|||
@ApiModelProperty(value = "所有人") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer userId; |
|||
|
|||
@ApiModelProperty(value = "是否删除, 0否, 1是") |
|||
@QueryField(type = QueryType.EQ) |
|||
private Integer deleted; |
|||
|
|||
} |
@ -0,0 +1,42 @@ |
|||
package com.gxwebsoft.tower.service; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.IService; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import com.gxwebsoft.tower.entity.TowerPartGoods; |
|||
import com.gxwebsoft.tower.param.TowerPartGoodsParam; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 安全检查Service |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 19:41:23 |
|||
*/ |
|||
public interface TowerPartGoodsService extends IService<TowerPartGoods> { |
|||
|
|||
/** |
|||
* 分页关联查询 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return PageResult<TowerPartGoods> |
|||
*/ |
|||
PageResult<TowerPartGoods> pageRel(TowerPartGoodsParam param); |
|||
|
|||
/** |
|||
* 关联查询全部 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return List<TowerPartGoods> |
|||
*/ |
|||
List<TowerPartGoods> listRel(TowerPartGoodsParam param); |
|||
|
|||
/** |
|||
* 根据id查询 |
|||
* |
|||
* @param goodsId 自增ID |
|||
* @return TowerPartGoods |
|||
*/ |
|||
TowerPartGoods getByIdRel(Integer goodsId); |
|||
|
|||
} |
@ -0,0 +1,42 @@ |
|||
package com.gxwebsoft.tower.service; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.IService; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import com.gxwebsoft.tower.entity.TowerPart; |
|||
import com.gxwebsoft.tower.param.TowerPartParam; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 安全检查Service |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 18:40:31 |
|||
*/ |
|||
public interface TowerPartService extends IService<TowerPart> { |
|||
|
|||
/** |
|||
* 分页关联查询 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return PageResult<TowerPart> |
|||
*/ |
|||
PageResult<TowerPart> pageRel(TowerPartParam param); |
|||
|
|||
/** |
|||
* 关联查询全部 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return List<TowerPart> |
|||
*/ |
|||
List<TowerPart> listRel(TowerPartParam param); |
|||
|
|||
/** |
|||
* 根据id查询 |
|||
* |
|||
* @param partId 自增ID |
|||
* @return TowerPart |
|||
*/ |
|||
TowerPart getByIdRel(Integer partId); |
|||
|
|||
} |
@ -0,0 +1,42 @@ |
|||
package com.gxwebsoft.tower.service; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.IService; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import com.gxwebsoft.tower.entity.TowerPartStock; |
|||
import com.gxwebsoft.tower.param.TowerPartStockParam; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 产品库存Service |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 19:41:23 |
|||
*/ |
|||
public interface TowerPartStockService extends IService<TowerPartStock> { |
|||
|
|||
/** |
|||
* 分页关联查询 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return PageResult<TowerPartStock> |
|||
*/ |
|||
PageResult<TowerPartStock> pageRel(TowerPartStockParam param); |
|||
|
|||
/** |
|||
* 关联查询全部 |
|||
* |
|||
* @param param 查询参数 |
|||
* @return List<TowerPartStock> |
|||
*/ |
|||
List<TowerPartStock> listRel(TowerPartStockParam param); |
|||
|
|||
/** |
|||
* 根据id查询 |
|||
* |
|||
* @param partId 自增ID |
|||
* @return TowerPartStock |
|||
*/ |
|||
TowerPartStock getByIdRel(Integer partId); |
|||
|
|||
} |
@ -0,0 +1,47 @@ |
|||
package com.gxwebsoft.tower.service.impl; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; |
|||
import com.gxwebsoft.tower.mapper.TowerPartGoodsMapper; |
|||
import com.gxwebsoft.tower.service.TowerPartGoodsService; |
|||
import com.gxwebsoft.tower.entity.TowerPartGoods; |
|||
import com.gxwebsoft.tower.param.TowerPartGoodsParam; |
|||
import com.gxwebsoft.common.core.web.PageParam; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 安全检查Service实现 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 19:41:23 |
|||
*/ |
|||
@Service |
|||
public class TowerPartGoodsServiceImpl extends ServiceImpl<TowerPartGoodsMapper, TowerPartGoods> implements TowerPartGoodsService { |
|||
|
|||
@Override |
|||
public PageResult<TowerPartGoods> pageRel(TowerPartGoodsParam param) { |
|||
PageParam<TowerPartGoods, TowerPartGoodsParam> page = new PageParam<>(param); |
|||
page.setDefaultOrder("create_time desc"); |
|||
List<TowerPartGoods> list = baseMapper.selectPageRel(page, param); |
|||
return new PageResult<>(list, page.getTotal()); |
|||
} |
|||
|
|||
@Override |
|||
public List<TowerPartGoods> listRel(TowerPartGoodsParam param) { |
|||
List<TowerPartGoods> list = baseMapper.selectListRel(param); |
|||
// 排序
|
|||
PageParam<TowerPartGoods, TowerPartGoodsParam> page = new PageParam<>(); |
|||
//page.setDefaultOrder("create_time desc");
|
|||
return page.sortRecords(list); |
|||
} |
|||
|
|||
@Override |
|||
public TowerPartGoods getByIdRel(Integer goodsId) { |
|||
TowerPartGoodsParam param = new TowerPartGoodsParam(); |
|||
param.setGoodsId(goodsId); |
|||
return param.getOne(baseMapper.selectListRel(param)); |
|||
} |
|||
|
|||
} |
@ -0,0 +1,47 @@ |
|||
package com.gxwebsoft.tower.service.impl; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; |
|||
import com.gxwebsoft.tower.mapper.TowerPartMapper; |
|||
import com.gxwebsoft.tower.service.TowerPartService; |
|||
import com.gxwebsoft.tower.entity.TowerPart; |
|||
import com.gxwebsoft.tower.param.TowerPartParam; |
|||
import com.gxwebsoft.common.core.web.PageParam; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 安全检查Service实现 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 18:40:31 |
|||
*/ |
|||
@Service |
|||
public class TowerPartServiceImpl extends ServiceImpl<TowerPartMapper, TowerPart> implements TowerPartService { |
|||
|
|||
@Override |
|||
public PageResult<TowerPart> pageRel(TowerPartParam param) { |
|||
PageParam<TowerPart, TowerPartParam> page = new PageParam<>(param); |
|||
//page.setDefaultOrder("create_time desc");
|
|||
List<TowerPart> list = baseMapper.selectPageRel(page, param); |
|||
return new PageResult<>(list, page.getTotal()); |
|||
} |
|||
|
|||
@Override |
|||
public List<TowerPart> listRel(TowerPartParam param) { |
|||
List<TowerPart> list = baseMapper.selectListRel(param); |
|||
// 排序
|
|||
PageParam<TowerPart, TowerPartParam> page = new PageParam<>(); |
|||
//page.setDefaultOrder("create_time desc");
|
|||
return page.sortRecords(list); |
|||
} |
|||
|
|||
@Override |
|||
public TowerPart getByIdRel(Integer partId) { |
|||
TowerPartParam param = new TowerPartParam(); |
|||
param.setPartId(partId); |
|||
return param.getOne(baseMapper.selectListRel(param)); |
|||
} |
|||
|
|||
} |
@ -0,0 +1,47 @@ |
|||
package com.gxwebsoft.tower.service.impl; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; |
|||
import com.gxwebsoft.tower.mapper.TowerPartStockMapper; |
|||
import com.gxwebsoft.tower.service.TowerPartStockService; |
|||
import com.gxwebsoft.tower.entity.TowerPartStock; |
|||
import com.gxwebsoft.tower.param.TowerPartStockParam; |
|||
import com.gxwebsoft.common.core.web.PageParam; |
|||
import com.gxwebsoft.common.core.web.PageResult; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 产品库存Service实现 |
|||
* |
|||
* @author 科技小王子 |
|||
* @since 2024-02-27 19:41:23 |
|||
*/ |
|||
@Service |
|||
public class TowerPartStockServiceImpl extends ServiceImpl<TowerPartStockMapper, TowerPartStock> implements TowerPartStockService { |
|||
|
|||
@Override |
|||
public PageResult<TowerPartStock> pageRel(TowerPartStockParam param) { |
|||
PageParam<TowerPartStock, TowerPartStockParam> page = new PageParam<>(param); |
|||
page.setDefaultOrder("create_time desc"); |
|||
List<TowerPartStock> list = baseMapper.selectPageRel(page, param); |
|||
return new PageResult<>(list, page.getTotal()); |
|||
} |
|||
|
|||
@Override |
|||
public List<TowerPartStock> listRel(TowerPartStockParam param) { |
|||
List<TowerPartStock> list = baseMapper.selectListRel(param); |
|||
// 排序
|
|||
PageParam<TowerPartStock, TowerPartStockParam> page = new PageParam<>(); |
|||
//page.setDefaultOrder("create_time desc");
|
|||
return page.sortRecords(list); |
|||
} |
|||
|
|||
@Override |
|||
public TowerPartStock getByIdRel(Integer partId) { |
|||
TowerPartStockParam param = new TowerPartStockParam(); |
|||
param.setPartId(partId); |
|||
return param.getOne(baseMapper.selectListRel(param)); |
|||
} |
|||
|
|||
} |
Loading…
Reference in new issue